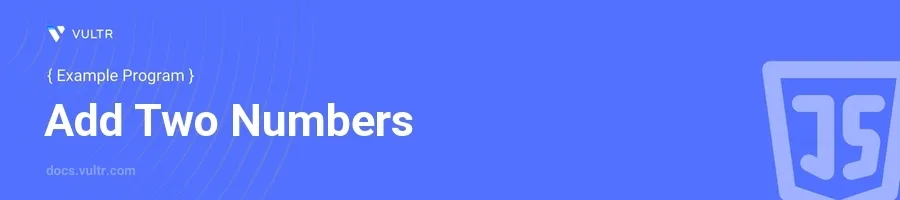
Introduction
Adding two numbers is one of the fundamental operations in programming, and JavaScript offers several ways to perform this task. Whether you're developing a web application, working on server-side scripting with Node.js, or simply practicing coding skills, understanding how to manipulate numbers is crucial.
In this article, you will learn how to add two numbers in JavaScript through various examples. This will include basic addition operations, adding numbers received from user input, and implementing addition within a function for reusability.
Basic Addition in JavaScript
Performing a Simple Add Operation
Assign values to two variables.
Use the
+
operator to add these variables.javascriptlet num1 = 5; let num2 = 10; let sum = num1 + num2; console.log(sum); // Output: 15
This code initializes two variables
num1
andnum2
with values5
and10
, respectively. The+
operator is then used to add these values, storing the result insum
. Finally, the result is printed to the console.
Addition Using User Inputs
Add Two Numbers Entered by the User
Use
prompt()
to get input from the user.Convert the input from strings to integers using
parseInt()
.javascriptlet num1 = parseInt(prompt("Enter the first number: ")); let num2 = parseInt(prompt("Enter the second number: ")); let sum = num1 + num2; alert("The sum is: " + sum);
This snippet uses the
prompt()
function to capture user inputs. Sinceprompt()
returns strings,parseInt()
is utilized to convert these strings to integers. The numbers are then added, and the result is displayed to the user viaalert()
.
Addition Inside a Function
Create a Function to Add Two Numbers
Define a function that takes two parameters.
Add the parameters and return the result.
javascriptfunction addNumbers(num1, num2) { return num1 + num2; } let result = addNumbers(15, 25); console.log("Sum obtained from the function: " + result);
Here,
addNumbers
is a function defined to take two parameters (num1
andnum2
). Inside the function, these parameters are added using the+
operator. The function returns the sum. When called with15
and25
as arguments, the function returns40
, which is then printed to the console.
Conclusion
Adding two numbers in JavaScript can be accomplished in a variety of ways, from simple arithmetic operations to handling user inputs and embedding addition logic within reusable functions. By mastering these basic numeric operations, you enhance your ability to handle more complex mathematical tasks in JavaScript applications. Utilize these examples to apply addition operations where necessary in your projects, ensuring that your application can perform essential calculations efficiently.
No comments yet.