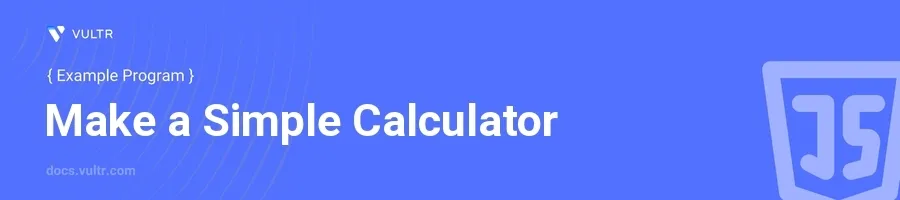
Introduction
A calculator program in JavaScript provides an excellent way to understand the fundamental concepts of programming like handling user input, executing arithmetic operations, and displaying results. Creating a simple calculator involves defining variables, reading user inputs, applying arithmetic formulas, and updating the webpage dynamically.
In this article, you will learn how to construct a basic calculator using JavaScript. Explore various examples, starting with basic arithmetic operations and progressing to the integration of these operations into a simple HTML interface.
Creating Basic Operations
Perform Addition
Define two numbers to add.
Sum the numbers using the
+
operator.Display the result in the console.
javascriptconst num1 = 5; const num2 = 3; const sum = num1 + num2; console.log('Sum:', sum);
This code sums
num1
andnum2
, then prints the result"Sum: 8"
to the console.
Perform Subtraction
Define two numbers.
Subtract the second number from the first.
Display the result in the console.
javascriptconst num1 = 10; const num2 = 6; const difference = num1 - num2; console.log('Difference:', difference);
This snippet subtracts
num2
fromnum1
and logs"Difference: 4"
to the console.
Perform Multiplication
Specify two numbers.
Multiply them using the
*
operator.Print the output.
javascriptconst num1 = 4; const num2 = 7; const product = num1 * num2; console.log('Product:', product);
The code calculates the product of
num1
andnum2
, resulting in"Product: 28"
.
Perform Division
Assign values to two variables.
Divide the first number by the second.
Log the result to the console.
javascriptconst num1 = 20; const num2 = 5; const quotient = num1 / num2; console.log('Quotient:', quotient);
This division operation yields a quotient of 4, as displayed in the console log.
Integrating Operations into a Web Interface
HTML Structure
Create a simple HTML form with input fields for the numbers and dropdown for selecting an operation.
Add a button to perform the calculation.
html<form> <input type="text" id="num1" placeholder="Enter First Number"> <input type="text" id="num2" placeholder="Enter Second Number"> <select id="operation"> <option value="add">Add</option> <option value="subtract">Subtract</option> <option value="multiply">Multiply</option> <option value="divide">Divide</option> </select> <button type="button" onclick="performCalculation()">Calculate</button> </form> <div id="result"></div>
JavaScript Implementation
Implement a function to fetch user inputs, perform the selected operation, and display the result.
javascriptfunction performCalculation() { const num1 = parseFloat(document.getElementById('num1').value); const num2 = parseFloat(document.getElementById('num2').value); const operation = document.getElementById('operation').value; let result; switch(operation) { case 'add': result = num1 + num2; break; case 'subtract': result = num1 - num2; break; case 'multiply': result = num1 * num2; break; case 'divide': result = num1 / num2; break; default: result = 'Invalid operation'; } document.getElementById('result').innerText = 'Result: ' + result; }
This script enables the calculator to process user input from the web form, calculate based on the chosen arithmetic operation, and display the result on the same page.
Conclusion
By creating a JavaScript program for a simple calculator, you gain a foundational understanding of handling user input, performing arithmetic operations, and dynamically updating web content. Start with basic arithmetic in standalone scripts and gradually integrate these into a functional web-based application. With this knowledge, modifying or expanding the calculator’s functionality becomes much more accessible, offering a solid starting point for more complex programming tasks.
No comments yet.