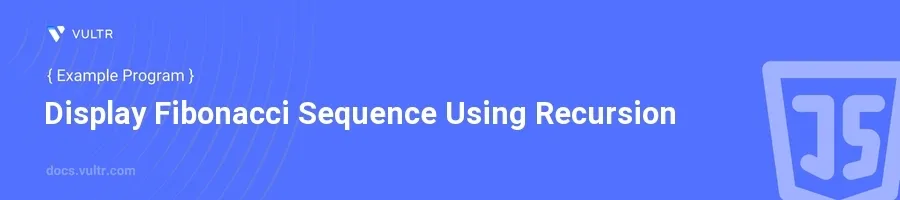
Introduction
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. It is a frequent subject of algorithmic problems and a good candidate for understanding recursive functions in programming. JavaScript is particularly suited for demonstrating concepts like recursion due to its straightforward syntax and function support.
In this article, you will learn how to create a JavaScript program to generate and display the Fibonacci sequence using recursion. You will examine a basic example and explore how the recursive mechanism computes each term of the sequence.
Understanding Recursion in JavaScript
Basic Concept of Recursion
Recursion in programming refers to a function calling itself during its execution. This self-reference is used for solving problems that can be broken down into smaller, repeated problem instances. For recursion to be properly used and not result in an infinite loop, it typically includes:
- A base case: the condition under which the function stops calling itself.
- A recursive case: the condition under which the function continues to call itself with modified arguments.
Constructing the Fibonacci Function
Define a function that accepts an index
n
.Implement a base case to identify when the sequence should stop recursively calling itself.
Use the recursive logic to continue the sequence until reaching the base case.
javascriptfunction fibonacci(n) { if (n < 2) { return n; } return fibonacci(n - 1) + fibonacci(n - 2); }
This function makes two recursive calls to itself, calculating Fibonacci numbers for
n-1
andn-2
until reaching the base cases wheren
is 0 or 1. In these cases, it directly returnsn
, as the Fibonacci sequence starts with0, 1
.
Displaying Fibonacci Sequence
To display the Fibonacci sequence up to a certain number in the sequence, wrap the recursion within a loop to generate each successive number.
Implementation to Display the Sequence
Decide the length of the Fibonacci sequence to display.
Iterate from 0 up to the chosen length, invoking the recursive function during each iteration.
Collect and print the result after each computation.
javascriptfunction displayFibonacci(sequenceLength) { for (let i = 0; i < sequenceLength; i++) { console.log(fibonacci(i)); } } displayFibonacci(10); // Display the first 10 Fibonacci numbers
In this code, the
displayFibonacci
function is responsible for determining how many numbers of the Fibonacci sequence to print. Inside the loop, thefibonacci
function is called with each index, and its result is logged to the console.
Conclusion
The Fibonacci sequence is a classical example to explore the power and elegance of recursive functions in JavaScript. By breaking down the problem into a base case and a recursive case, it’s possible to generate complex sequences with a minimal amount of simple, readable code. With this knowledge, decide where you might apply recursion in your own JavaScript projects, especially in tasks involving iterative computations or complex chained processes. Remember that while recursion offers a neat solution, it can also lead to performance issues if overused or used improperly, so always consider the alternatives such as iterative methods or dynamic programming techniques.
No comments yet.