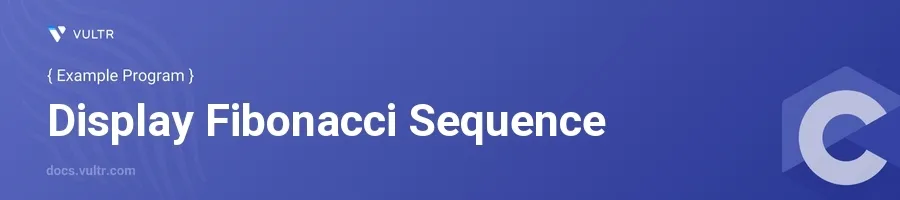
Introduction
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. It's a famous sequence that appears in many areas of mathematics and science and is often used as a classic programming exercise to introduce iterative and recursive functions.
In this article, you will learn how to implement a C program to display the Fibonacci sequence. You will explore both iterative and recursive methods through detailed examples, enabling you to understand the strengths and limitations of each approach.
Iterative Approach to Fibonacci Sequence
Display Fibonacci Sequence Using For Loop
Initialize the sequence with the first two numbers.
Iterate through the desired number of elements, updating the sequence in each iteration.
c#include <stdio.h> void printFibonacciIterative(int n) { int t1 = 0, t2 = 1, nextTerm; printf("Fibonacci Series: %d, %d", t1, t2); for (int i = 3; i <= n; i++) { nextTerm = t1 + t2; printf(", %d", nextTerm); t1 = t2; t2 = nextTerm; } } int main() { int n = 10; // Display the first 10 Fibonacci numbers printFibonacciIterative(n); return 0; }
This code snippet initializes the first two terms of the Fibonacci sequence. It then enters a loop, generating the next term by summing the two previous terms and updates them for the next iteration. The sequence generated will be the first
n
elements of the sequence.
Recursive Approach to Fibonacci Sequence
Calculate Fibonacci Sequence Using Recursion
Define a recursive function that returns the nth Fibonacci number.
Use the recursive function in the main program to display the sequence.
c#include <stdio.h> int fibonacciRecursive(int n) { if (n <= 1) { return n; } return fibonacciRecursive(n - 1) + fibonacciRecursive(n - 2); } int main() { int n = 10; // Number of terms to display printf("Fibonacci Sequence: "); for (int i = 0; i < n; i++) { printf("%d, ", fibonacciRecursive(i)); } return 0; }
This code defines a function
fibonacciRecursive
which calculates the Fibonacci number using recursion. The function calls itself to get the two preceding Fibonacci numbers until it reaches the base case (either 0 or 1). The main function uses this recursive function in a loop to print each Fibonacci number in sequence.
Conclusion
Implementing the Fibonacci sequence in C demonstrates the basic concepts of loops and recursion. The iterative approach is generally more efficient for calculating Fibonacci numbers as it does not involve the overhead of multiple function calls that occur in recursion. However, the recursive method provides a clear and direct mapping to the mathematical definition of the sequence. Choose the method that best suits your needs, understanding both the simplicity of the iterative method and the elegance of the recursive approach. By mastering both methods, gain a deeper understanding of how different algorithms can solve the same problem in programming.
No comments yet.