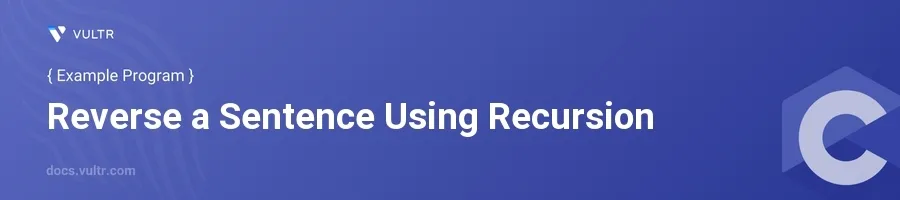
Introduction
Reversing a sentence in C programming using recursion is an excellent exercise to understand the fundamentals of strings and recursive functions. This approach not only strengthens your grasp on C syntax but also improves your problem-solving skills by utilizing the concept of recursion where a function calls itself to perform a task.
In this article, you will learn how to craft a simple C program that can reverse a sentence using recursion. You will go through the setup of the recursive function and see examples of how it works in practice.
Setting Up the C Program
Define the Recursive Function
Start by including the necessary header files.
Declare a recursive function that takes a string (character array) as its argument.
Use recursion within the function to reverse the sentence.
c#include <stdio.h> void reverseSentence() { char c; scanf("%c", &c); if (c != '\n') { reverseSentence(); printf("%c", c); } }
In this code, the function
reverseSentence
reads a character from the input until it encounters a newline. When the newline character is detected, the function starts to unwind and prints the characters in reverse order as it returns from each recursive call.
Implement the Main Function
In the
main()
function, prompt the user to enter a sentence.Call the recursive function to reverse the sentence.
Add a return statement to signify the end of the program.
cint main() { printf("Enter a sentence: "); reverseSentence(); return 0; }
This main function sets the stage by asking for user input. After the sentence is input, the
reverseSentence
function is invoked to perform the reversal using recursion.
Example Usage
Here is how the program works in practice:
- If the user inputs the sentence "Hello, World!", the program displays "!dlroW ,olleH" as output.
Running this program in a C compiler will demonstrate the reversed sentence, showcasing the practical application of recursion in string manipulation.
Conclusion
The reverseSentence
function in this C program demonstrates a fundamental use of recursion to reverse a sentence. By entering into deeper levels of function calls with each character and unwinding those calls in the opposite order, the program effectively reverses the order of characters in the sentence. Utilize this recursive approach to enhance your understanding and manipulation of strings in C programming, turning complex problems into manageable tasks with elegant solutions.
No comments yet.