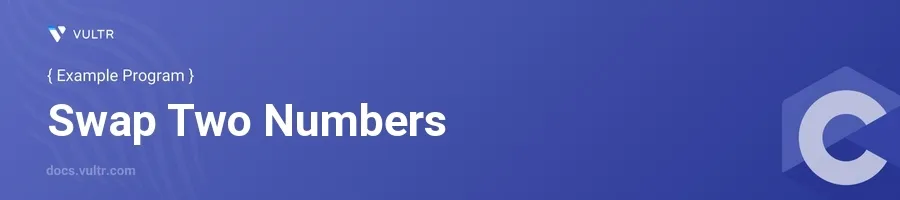
Introduction
Swapping two numbers is a fundamental operation in programming that entails trading the values held by two variables. This process is often used in sorting algorithms, permutation generating algorithms, and where resource optimization is needed by bypassing temporary holders.
In this article, you will learn how to effectively perform the number swapping operation in C programming through various methods. Get insights on using both temporary variables and arithmetic operations to achieve this, enhancing your understanding of variable manipulation in programming.
Swapping Using a Temporary Variable
Basic Swap Mechanism
Define two integer variables with initial values.
Declare a temporary variable to aid in the swap process.
Perform the swap using the temporary variable.
c#include <stdio.h> int main() { int a = 5, b = 10, temp; temp = a; a = b; b = temp; printf("After swap: a = %d, b = %d\n", a, b); return 0; }
Here, the
temp
variable holds the value ofa
temporarily whilea
gets the value ofb
. Then,b
is assigned the value oftemp
, successfully swapping the values ofa
andb
.
Swapping Without a Temporary Variable
Using Arithmetic Operations
Initialize two integers.
Use addition and subtraction to swap values without a temporary variable.
c#include <stdio.h> int main() { int a = 5, b = 10; a = a + b; // a now contains both a and b b = a - b; // subtract b from the new a, giving original a a = a - b; // subtract new b from the new a, giving original b printf("After swap: a = %d, b = %d\n", a, b); return 0; }
This method involves the variables
a
andb
directly engaging in arithmetic operations that result in their values being swapped. Note that this approach may cause overflow if the integer limits are exceeded.
Using Bitwise XOR Operation
Start with initializing two integers.
Apply the XOR operation to swap the numbers without using extra space.
c#include <stdio.h> int main() { int a = 5, b = 10; a = a ^ b; b = a ^ b; a = a ^ b; printf("After swap: a = %d, b = %d\n", a, b); return 0; }
The XOR bitwise operation here exploits the properties of XOR to swap the numbers efficiently. It's a particularly clever trick for memory-constrained environments since no additional storage is required.
Conclusion
Swapping variables efficiently in C can be achieved in several ways, each suitable for different contexts and performance needs. Whether using a traditional temporary variable approach, leveraging arithmetic operations, or employing bitwise manipulations, understand the implications and limitations of each to utilize them effectively in your coding tasks. Optimizing variable swapping can lead to more efficient code, especially in algorithms that rely heavily on element repositioning such as sorting algorithms.
No comments yet.