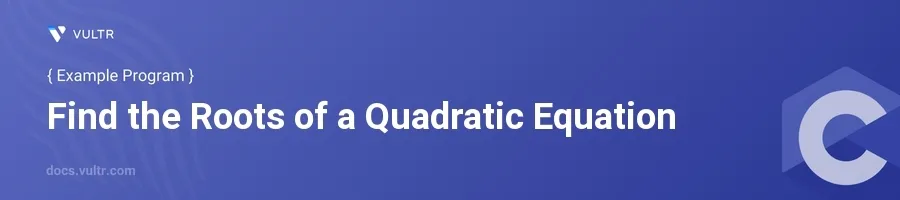
Introduction
Determining the roots of a quadratic equation is a fundamental problem in algebra, commonly solved in many engineering and scientific computations. In programming, particularly in C, solving this equation involves understanding not only the mathematical concepts but also how to implement them using the language's syntax and functions.
In this article, you will learn how to write a C program to find the roots of a quadratic equation. You'll explore setting up the equation, using the quadratic formula, and handling different types of roots—real, complex, and repeating.
Crafting the Basic Structure
Setup Your C Program
Start with including the necessary header files. Use
stdio.h
for input-output operations andmath.h
for mathematical functions.Declare the main function.
c#include <stdio.h> #include <math.h> int main() { // Your code will go here return 0; }
This structure sets up a C program by including headers for the I/O and mathematical operations, and declares the main function from where the execution starts.
Define Variables
Declare variables to store the coefficients of the quadratic equation (
a
,b
,c
) and the roots (root1
,root2
).cdouble a, b, c, root1, root2;
This line of code defines double type variables to store float values representing coefficients and roots which can be either real or imaginary.
Implementing the Quadratic Formula
Input the Coefficients
Prompt the user to input the values of
a
,b
, andc
.cprintf("Enter coefficients a, b, and c: "); scanf("%lf %lf %lf", &a, &b, &c);
Here, values entered by the user are stored in the variables
a
,b
, andc
usingscanf
.
Compute the Discriminant
Calculate the discriminant to determine the nature of the roots using the formula ( D = b^2 - 4ac ).
cdouble discriminant = b * b - 4 * a * c;
Discriminant helps in deciding whether the roots are real, imaginary, or equal.
Calculate and Display the Roots
Use conditional statements to compute the roots based on the discriminant's value.
cif (discriminant > 0) { root1 = (-b + sqrt(discriminant)) / (2 * a); root2 = (-b - sqrt(discriminant)) / (2 * a); printf("Roots are real and different: %.2lf and %.2lf\n", root1, root2); } else if (discriminant == 0) { root1 = root2 = -b / (2 * a); printf("Roots are real and equal: %.2lf\n", root1); } else { double realPart = -b / (2 * a); double imagPart = sqrt(-discriminant) / (2 * a); printf("Roots are complex: %.2lf+%.2lfi and %.2lf-%.2lfi\n", realPart, imagPart, realPart, imagPart); }
Depending on the discriminant value, the program calculates either two distinct real roots, one repeated real root, or two complex conjugate roots.
Conclusion
Writing a C program to solve quadratic equations lets you practice handling input, mathematical computation, and conditional logic—all of which are crucial for software development. With the program discussed, handle any quadratic equations by calculating and distinguishing between different types of roots, advancing your ability to tackle more complex problems using C. Ensure all input coefficients are valid to avoid runtime errors and ensure accurate computation of roots.
No comments yet.