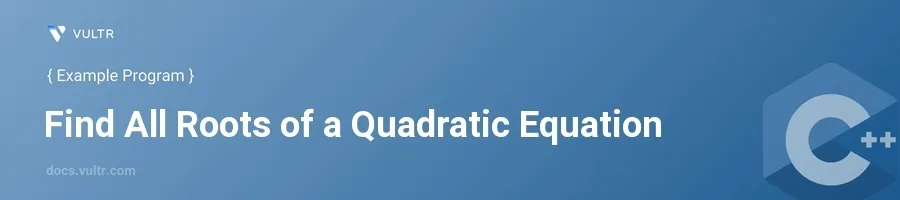
Introduction
Quadratic equations are fundamental in algebra and appear frequently in various mathematical problems. They have the standard form ( ax^2 + bx + c = 0 ), where ( a ), ( b ), and ( c ) are coefficients and ( x ) represents the unknown variable. Solving these equations involves finding the values of ( x ) that satisfy the equation, commonly known as the roots of the equation.
In this article, you will learn how to create a C++ program to find all roots of a quadratic equation. Explore different methodologies for solving quadratic equations programmatically, including handling real and complex roots. Implementations and examples will guide you through using conditionals and mathematical functions to compute and display the roots effectively.
Setting Up Your C++ Environment
Before diving into the coding part, ensure you have an environment set up for compiling and running C++ programs. You might use an integrated development environment (IDE) like Visual Studio, Code::Blocks, or simple text editors combined with a command-line compiler like GCC or Clang.
Implementing Root Calculation in C++
Identifying the Types of Roots
- Understand the discriminant ( \Delta ) which is ( b^2 - 4ac ). The nature of the roots is determined by the value of the discriminant:
- If ( \Delta > 0 ), the equation has two distinct real roots.
- If ( \Delta = 0 ), the equation has exactly one real root (also called a repeated or double root).
- If ( \Delta < 0 ), the equation has two complex roots.
Writing the Code to Find the Roots
Begin by including the necessary headers and using the standard namespace.
Declare the main function.
cpp#include <iostream> #include <cmath> // Include cmath for the sqrt() function using namespace std; int main() { double a, b, c, discriminant, root1, root2, realPart, imagPart;
Prompt the user to enter the coefficients ( a ), ( b ), and ( c ) and store these values.
Calculate the discriminant to decide the nature of the roots.
Use conditional statements to calculate and print the roots based on the value of the discriminant.
cppcout << "Enter coefficients a, b and c: "; cin >> a >> b >> c; discriminant = b*b - 4*a*c; if (discriminant > 0) { // Two distinct and real roots root1 = (-b + sqrt(discriminant)) / (2*a); root2 = (-b - sqrt(discriminant)) / (2*a); cout << "Roots are real and different." << endl; cout << "Root 1 = " << root1 << endl; cout << "Root 2 = " << root2 << endl; } else if (discriminant == 0) { // One double or repeated root root1 = root2 = -b / (2*a); cout << "Roots are real and same." << endl; cout << "Root 1 = Root 2 = " << root1 << endl; } else { // Complex and imaginary roots realPart = -b/(2*a); imagPart = sqrt(-discriminant)/(2*a); cout << "Roots are complex and different." << endl; cout << "Root 1 = " << realPart << "+" << imagPart << "i" << endl; cout << "Root 2 = " << realPart << "-" << imagPart << "i" << endl; } return 0; }
In this snippet:
- The program calculates the discriminant to identify the type of roots.
- Based on the discriminant, appropriate roots are calculated.
- Roots are either real (and the same or different) or complex.
- Results are printed directly to the console.
Handling Edge Cases
To ensure robustness:
- Validate input by checking that ( a \neq 0 ) since ( a = 0 ) would not represent a quadratic equation but a linear one.
- Consider implementing error handling for non-numeric inputs which might cause your program to break or behave unexpectedly.
Conclusion
Understanding how to determine and calculate the roots of quadratic equations in C++ equips you with fundamental skills that apply in various mathematical and technical domains. By following the examples shown, you handle different scenarios a quadratic equation might present, ensuring your program can compute both real and complex roots accurately. This knowledge aids in tasks ranging from simple homework problems to complex physics simulations where such equations are common.
No comments yet.