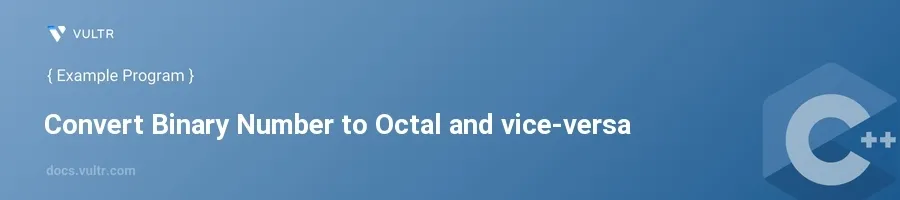
Introduction
Handling different numeral systems efficiently is a fundamental skill in programming, especially when dealing with low-level operations or systems programming. C++ provides a robust set of tools and functions that make these conversions straightforward. Converting between binary and octal numeral systems is particularly common due to their direct relationship, each being powers of 2 (2^2 for binary and 2^3 for octal), which simplifies the conversion process.
In this article, you will learn how to convert binary numbers to octal numbers and vice versa using C++. Explore practical examples that detail each step of the conversion process, ensuring that you can implement these methods in your own C++ applications.
Converting Binary to Octal
Understanding the Relationship
- Recognize that each octal digit directly corresponds to three binary digits.
- This correlation allows grouping the binary digits in sets of three, starting from the right (least significant bits).
Conversion Steps
Start with a binary number as input.
Check if the length of the binary number is a multiple of three. If not, pad the number on the left with zeros.
cpp#include <iostream> #include <string> std::string padBinary(std::string binary) { while (binary.length() % 3 != 0) { binary = "0" + binary; } return binary; }
This function adds leading zeros to the binary string until its length is a multiple of three.
Group the binary number into sets of three digits.
Convert each group to its corresponding octal digit.
cppchar binaryToOctalDigit(std::string binaryThreeDigit) { if (binaryThreeDigit == "000") return '0'; else if (binaryThreeDigit == "001") return '1'; else if (binaryThreeDigit == "010") return '2'; // Add remaining cases as needed else if (binaryThreeDigit == "111") return '7'; return '0'; // Default case, should not happen } std::string binaryToOctal(std::string binary) { binary = padBinary(binary); std::string octal = ""; for (size_t i = 0; i < binary.length(); i += 3) { octal += binaryToOctalDigit(binary.substr(i, 3)); } return octal; }
This function first pads the binary string, then converts each triplet of binary digits to the corresponding octal digit.
Converting Octal to Binary
Conversion Steps
Start with an octal number as input.
Convert each octal digit into its binary equivalent, ensuring each binary group has exactly three digits.
cppstd::string octalDigitToBinary(char octalDigit) { switch (octalDigit) { case '0': return "000"; case '1': return "001"; case '2': return "010"; case '3': return "011"; case '4': return "100"; case '5': return "101"; case '6': return "110"; case '7': return "111"; default: return "000"; // Default case, should not happen } } std::string octalToBinary(std::string octal) { std::string binary = ""; for (char digit : octal) { binary += octalDigitToBinary(digit); } return binary; }
Here, each digit of the octal string is converted to a binary string of three digits.
Conclusion
Efficiently converting between binary and octal numbers in C++ can significantly enhance the performance and versatility of your applications, especially in fields requiring numerical transformations. By mastering these conversion techniques, you equip yourself with the necessary tools to handle various numeral systems with ease. Utilize these methods to maintain clear, efficient, and effective code when dealing with binary and octal numbers in your projects.
No comments yet.