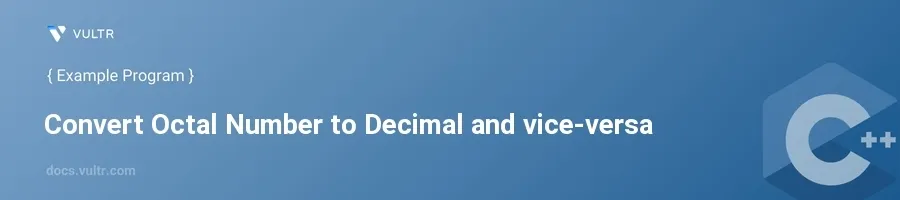
Introduction
Octal and decimal number systems play a crucial role in computer science, particularly in fields related to computing and digital electronics. While the decimal system is base-10, using digits from 0 to 9, the octal system is base-8, using digits from 0 to 7. Being able to convert between these systems is essential for certain low-level programming tasks and understanding computer architecture.
In this article, you will learn how to write C++ programs that convert numbers from octal to decimal and vice-versa. Explore practical examples that demonstrate each conversion process, ensuring you can integrate this knowledge effectively in your projects or enhance your understanding of these number systems.
Octal to Decimal Conversion
Conversion from octal to decimal involves understanding the place value of each digit in the octal number and converting it to its decimal equivalent by summing the products of each digit with eight raised to the power of the digit's position (starting from 0).
Example: Converting an Octal Number to Decimal
Define the octal number.
Initialize a decimal result variable.
Process each digit of the octal number.
cpp#include <iostream> #include <cmath> // For pow function int octalToDecimal(int octal) { int decimal = 0, i = 0; while (octal != 0) { int digit = octal % 10; decimal += digit * pow(8, i); ++i; octal /= 10; } return decimal; } int main() { int octalNumber = 123; int decimalNumber = octalToDecimal(octalNumber); std::cout << "Decimal: " << decimalNumber << std::endl; return 0; }
This function takes an octal number as input, extracts each digit starting from the least significant (rightmost), multiplies it by
8
raised to a power incrementing from0
, and adds this to a cumulative sum to get the decimal equivalent.
Decimal to Octal Conversion
Converting a decimal number to octal involves determining the remainder of the number when divided by 8
. This process repeats for the quotient until the quotient becomes zero. The remainders collected form the octal number in reverse order.
Example: Converting a Decimal Number to Octal
Define the decimal number.
Initialize an octal result and a factor to manage the powers of 10.
Convert in a loop by obtaining remainders and adjusting the base.
cpp#include <iostream> int decimalToOctal(int decimal) { int octal = 0, factor = 1; while (decimal != 0) { int remainder = decimal % 8; octal += remainder * factor; factor *= 10; decimal /= 8; } return octal; } int main() { int decimalNumber = 83; int octalNumber = decimalToOctal(decimalNumber); std::cout << "Octal: " << octalNumber << std::endl; return 0; }
This function processes the decimal number by continuously dividing it by
8
, capturing remainders. These remainders form the octal number when combined in the reverse order of derivation.
Conclusion
The code examples provided illustrate how to convert numbers between octal and decimal systems using C++. These conversions are crucial for applications involving low-level programming or systems where octal notation is preferred for its compactness relative to binary. Leverage these conversion techniques in your applications where handling different numeral systems is required. With practice, integrate these conversions smoothly to handle computational tasks more efficiently.
No comments yet.