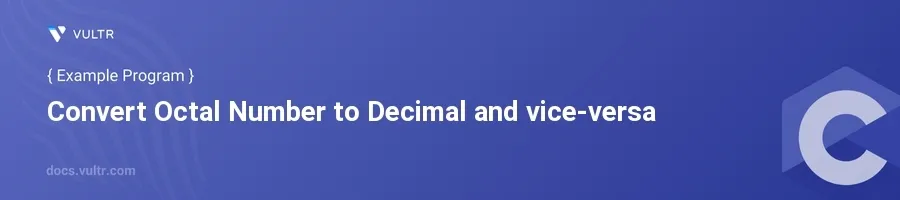
Introduction
Octal and decimal number systems are two different bases that are commonly used in computing—octal (base-8) and decimal (base-10). Understanding how to convert between these systems is not only crucial for computer science studies but also essential when dealing with low-level programming or computer architecture.
In this article, you will learn how to write C programs to convert octal numbers to decimal and vice versa. Through detailed examples, you'll gain insights into how each conversion process works and how you can implement these conversions efficiently using C.
Converting Octal to Decimal
Understand the Conversion Process
The conversion from octal to decimal involves processing each digit of the octal number starting from the rightmost digit and moving left. Each digit is multiplied by (8^n) (where (n) is the position of the digit from right to left, starting at 0), and the results are summed to obtain the decimal equivalent.
Example: Convert an Octal Number to a Decimal Number
Take an octal number as input.
Initialize
decimal_value
to 0 andbase
to 1 (which is (8^0)).c#include <stdio.h> int main() { char octalNum[100]; int decimalValue = 0, base = 1; int length, i, value; printf("Enter an octal number: "); scanf("%s", octalNum); for(length = 0; octalNum[length]!='\0'; length++); for(i = length - 1; i >= 0; i--) { if(octalNum[i] >= '0' && octalNum[i] <= '7') { value = octalNum[i] - '0'; decimalValue += value * base; base *= 8; } else { printf("Invalid octal number.\n"); return -1; } } printf("Decimal value: %d\n", decimalValue); return 0; }
Here, the code reads an octal number as a string and processes each digit from right to left. If the digit is valid (0 through 7), it converts it to its decimal equivalent and sums it up. Otherwise, it issues an error message.
Converting Decimal to Octal
Understand the Conversion Process
To convert a decimal number to its octal equivalent, repeatedly divide the number by 8 and record the remainder for each division in reverse order until the number becomes 0.
Example: Convert a Decimal Number to an Octal Number
Take a decimal number as input.
Use an array to store octal digits.
c#include <stdio.h> int main() { int decimalNum, remainder, i = 1, j; int octalNum[100]; printf("Enter a decimal number: "); scanf("%d", &decimalNum); while(decimalNum != 0) { remainder = decimalNum % 8; octalNum[i++] = remainder; decimalNum /= 8; } printf("Octal value: "); for(j = i - 1; j > 0; j--) printf("%d", octalNum[j]); printf("\n"); return 0; }
This code collects the remainders of the divisons by 8 in an array and then prints them in reverse order to display the octal number.
Conclusion
The conversion between octal and decimal formats in C can be performed efficiently using simple arithmetic operations. These conversions are not just academic exercises but are also used in applications such as data manipulation and system programming. By mastering these conversions, ensure your programs can handle different numbering systems with ease, enhancing both their versatility and performance.
No comments yet.