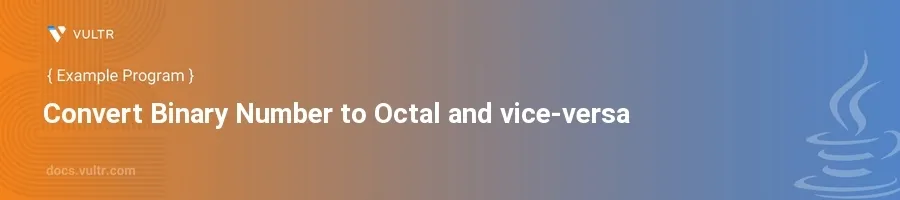
Introduction
Java offers multiple ways to manipulate and convert numbers between different bases, such as binary and octal, which are essential for various computing tasks and understanding computer architecture. Converting numbers between these bases can also be handy in applications involving low-level programming or systems design where different numeral systems are more appropriate.
In this article, you learn how to create Java programs to convert a binary number to an octal number and vice versa. These examples, including binary to octal in java, will enhance your understanding of numeral systems and Java's capabilities in handling such conversions effectively.
Converting Binary to Octal in Java
Understanding the Conversion Process
To convert a binary number (base 2) to an octal number (base 8), follow these steps:
- Convert the binary number to a decimal (base 10) as an intermediary step.
- Convert the decimal number into octal.
Java Implementation
Create a method to convert binary to decimal.
Create another method to convert decimal to octal.
Combine both methods to perform the binary to octal conversion.
javapublic class BinaryToOctal { public static int binaryToDecimal(String binary) { return Integer.parseInt(binary, 2); } public static String decimalToOctal(int decimal) { return Integer.toOctalString(decimal); } public static void main(String[] args) { String binary = "101101"; int decimal = binaryToDecimal(binary); String octal = decimalToOctal(decimal); System.out.println("Binary to Octal: " + octal); } }
This code first converts a binary string to a decimal integer using
Integer.parseInt()
with base 2. Then, it converts that decimal to an octal string usingInteger.toOctalString()
. The result is printed to the console.
Converting Octal to Binary In Java
Understanding the Conversion Process
To reverse the process, converting an octal number back to binary involves:
- Converting the octal number to a decimal.
- Converting the decimal number to binary.
Java Implementation
Create a method to convert octal to decimal.
Create another method to convert decimal to binary.
Combine both methods to perform the octal to binary conversion.
javapublic class OctalToBinary { public static int octalToDecimal(String octal) { return Integer.parseInt(octal, 8); } public static String decimalToBinary(int decimal) { return Integer.toBinaryString(decimal); } public static void main(String[] args) { String octal = "55"; int decimal = octalToDecimal(octal); String binary = decimalToBinary(decimal); System.out.println("Octal to Binary: " + binary); } }
In this snippet, the
Integer.parseInt()
method takes an octal string with base 8 to convert it to a decimal integer. Then,Integer.toBinaryString()
is used to convert the decimal integer to a binary string. The result is displayed in the console.
Conclusion
The Java programming language provides robust methods for number conversions between different bases, making tasks like binary to octal conversion in java straightforward. Utilize these methods to handle similar tasks in your projects or to deepen your understanding of how data is formatted and manipulated at a low level. Through these examples, you ensure precision and efficiency in number handling in Java applications.
No comments yet.