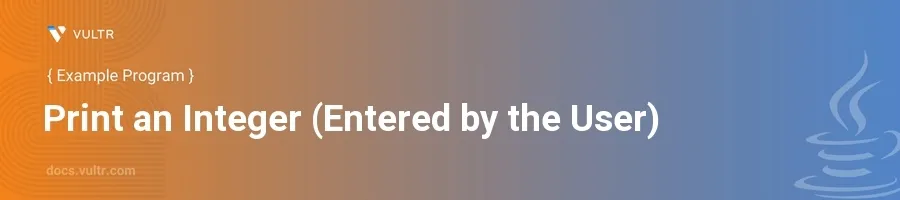
Introduction
Java is a robust programming language widely used for building enterprise-scale applications, Android apps, and much more. One fundamental concept in any programming language is handling user inputs and displaying outputs. This forms the foundation of interactive applications.
In this article, you will learn how to create a Java program that prompts the user to enter an integer and then prints it. Explore different examples illustrating how to implement this simple but essential task using various Java methods.
Using Scanner for User Input
Basic Integer Input and Output
Import the
Scanner
class which is used to read data entered by the user.Create an instance of
Scanner
.Prompt the user to enter an integer.
Use the
nextInt()
method to read the integer.Print the integer entered by the user.
javaimport java.util.Scanner; public class IntegerPrinter { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Enter an integer: "); int number = scanner.nextInt(); System.out.println("You entered: " + number); } }
This code snippet creates a
Scanner
object that reads integers from the standard input (keyboard). The program then usesSystem.out.println
to display the integer that the user entered.
Handling Input Exceptions
Implement error handling to manage non-integer inputs.
Use a
try
block to attempt reading an integer.Catch a potential
InputMismatchException
if a non-integer is entered.Prompt the user again for an integer.
javaimport java.util.InputMismatchException; import java.util.Scanner; public class IntegerPrinter { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Enter an integer: "); try { int number = scanner.nextInt(); System.out.println("You entered: " + number); } catch (InputMismatchException e) { System.out.println("That's not an integer. Please enter an integer."); } } }
This enhanced version of the program includes exception handling. If the user inputs something that isn’t an integer, the
InputMismatchException
is caught, and a relevant message is displayed.
Using JOptionPane for Graphical User Input
Using JOptionPane for Input and Output
Import the
JOptionPane
class for using GUI elements.Use
JOptionPane.showInputDialog()
to get user input through a dialog box.Parse the input string to an integer.
Display the integer using
JOptionPane.showMessageDialog()
.javaimport javax.swing.JOptionPane; public class IntegerPrinterGUI { public static void main(String[] args) { String input = JOptionPane.showInputDialog(null, "Enter an integer:"); try { int number = Integer.parseInt(input); JOptionPane.showMessageDialog(null, "You entered: " + number); } catch (NumberFormatException e) { JOptionPane.showMessageDialog(null, "That's not an integer. Please enter an integer."); } } }
This version uses the
JOptionPane
class to create graphical dialogs for both input and output. It's a more user-friendly way and suitable for GUI applications but requires thejavax.swing
library.
Conclusion
Creating a Java program to print an integer entered by a user demonstrates the basic skills of handling inputs and outputs in Java. Starting with the console-based input using Scanner
and advancing to graphical input with JOptionPane
provides you with the flexibility to implement user interaction in both console applications and GUIs. By mastering these methods, you ensure your Java applications can interact effectively with users, enhancing both functionality and user experience.
No comments yet.