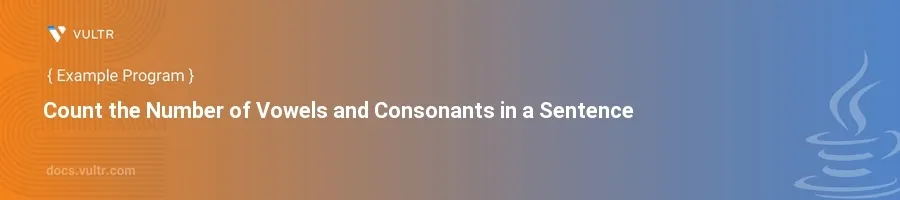
Introduction
When working with text in Java, identifying the number of vowels and consonants within a sentence can provide valuable insights into the content's structure. This sort of analysis can be used in various applications, from formulating educational tools to natural language processing.
In this article, you will learn how to develop a Java program that counts the number of vowels and consonants in a given sentence. You will explore several examples demonstrating different techniques to achieve this, emphasizing practical application and efficiency.
Count Using Loop and Conditional Statements
Basic Approach Using For Loop
Initialize counters for vowels and consonants to zero.
Convert the sentence to lowercase to make the program case-insensitive.
Use a for loop to iterate through each character in the sentence.
Within the loop, use conditional statements to check whether the character is a vowel or consonant and increment the corresponding counters.
javapublic class VowelConsonantCounter { public static void main(String[] args) { String sentence = "Example sentence goes here."; int vowels = 0, consonants = 0; String lowerSentence = sentence.toLowerCase(); for (int i = 0; i < lowerSentence.length(); i++) { char ch = lowerSentence.charAt(i); if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u') { vowels++; } else if ((ch >= 'a' && ch <= 'z')) { consonants++; } } System.out.println("Number of vowels: " + vowels); System.out.println("Number of consonants: " + consonants); } }
The code iterates through the sentence, checking each character to determine if it is a vowel or consonant. Non-alphabetic characters are ignored.
Using Stream API
Stream Filters and Lambdas
Use Java's Stream API for a more modern approach.
Convert the sentence into a stream of characters.
Apply filters to count vowels and consonants separately.
javaimport java.util.Arrays; public class VowelConsonantCounterStream { public static void main(String[] args) { String sentence = "Example sentence goes here."; long vowels = sentence.toLowerCase().chars() .filter(ch -> "aeiou".indexOf(ch) >= 0) .count(); long consonants = sentence.toLowerCase().chars() .filter(ch -> ch >= 'a' && ch <= 'z') .filter(ch -> "aeiou".indexOf(ch) < 0) .count(); System.out.println("Number of vowels: " + vowels); System.out.println("Number of consonants: " + consonants); } }
In this example, the sentence is converted to a stream of characters, and filters are applied to count vowels and consonants. This approach is succinct and leverages the powerful Stream API for processing sequences of elements.
Conclusion
Counting vowels and consonants in a sentence in Java can be accomplished through traditional loop and conditional operations or through the use of Java's Stream API. Select the approach that best fits the context of your project; traditional methods offer straightforward logic that is easy to understand for beginners, while the Stream API provides a more elegant and concise solution suitable for larger datasets or more complex applications. Apply these methods to expand your Java programming skills and incorporate textual analysis into your applications.
No comments yet.