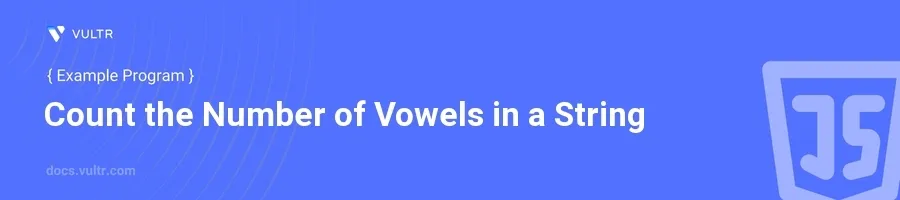
Introduction
Counting the number of vowels in a string is a common problem that can be solved using JavaScript. This task is not only fundamental in learning how string manipulation works but also serves as a great example to understand loops, conditionals, and basic function creation in JavaScript. With this program you will learn how to make code count vowels in a string using JavaScript .
In this article, you will learn how to create a JavaScript program to count the number of vowels in a string. You'll see different methods, including both iterative and functional approaches, to perform this task, allowing you to understand the flexibility and power of JavaScript for simple data processing tasks like these.
Defining the Vowel Counting Function
Using a For Loop to write a JavaScript code to count number of vowels in string using a function
Start by defining a function that accepts a string as an argument.
Initialize a counter to zero to keep track of the number of vowels.
Loop through each character in the string.
Check if the character is a vowel by comparing it against a set of vowels.
If it's a vowel, increment the counter.
Return the counter after the loop ends.
javascriptfunction countVowels(str) { const vowels = "aeiouAEIOU"; let count = 0; for (let i = 0; i < str.length; i++) { if (vowels.includes(str[i])) { count++; } } return count; }
In this code snippet, the function
countVowels
checks every character in the input string to determine if it is a vowel by seeing if it's included in the stringvowels
. Theincludes
method returnstrue
if the character is found, hence, the counter is incremented. This approach is commonly used to count vowels in a string in JavaScript, ensuring an efficient way to process text.
Using the filter()
Array Method
Define a function to count vowels using array and functional programming techniques.
Convert the string to an array of characters.
Use the
filter()
method to sift out the vowels.Return the length of the array formed by
filter()
.javascriptfunction countVowelsFP(str) { const vowels = "aeiouAEIOU"; return Array.from(str).filter(char => vowels.includes(char)).length; }
Here,
Array.from(str)
creates an array from the input string, andfilter()
creates a new array containing only the characters that meet the condition specified in its argument. The condition being checking against thevowels
string. Finally, the length of the resulting array gives the count of vowels in a string using JavaScript.
Case Insensitivity and Special Characters
Enhance your function to handle case insensitivity by converting the entire string to lower or upper case before counting.
Ensure also that only alphabetical characters are counted to avoid errors with special characters or numbers.
javascriptfunction countVowelsEnhanced(str) { const vowels = "aeiou"; str = str.toLowerCase(); let count = 0; for (let char of str) { if (vowels.includes(char) && char >= 'a' && char <= 'z') { count++; } } return count; }
This enhanced function ensures that the comparison is case-insensitive by converting the string to lowercase with
toLowerCase()
. It also makes a rudimentary check that the character is indeed a letter.
Conclusion
Counting vowels in a string using JavaScript provides a good exercise in string manipulation, iteration, and functional programming concepts. Whether you choose a straightforward loop method or a more functional programming approach with filter()
, JavaScript offers multiple avenues to solve the problem efficiently. Employ these methods to handle various string processing tasks, enriching your JavaScript coding skills for both practical applications and algorithmic challenges. By mastering these techniques, you ensure that your code is not only functional but also clean and efficient.
No comments yet.