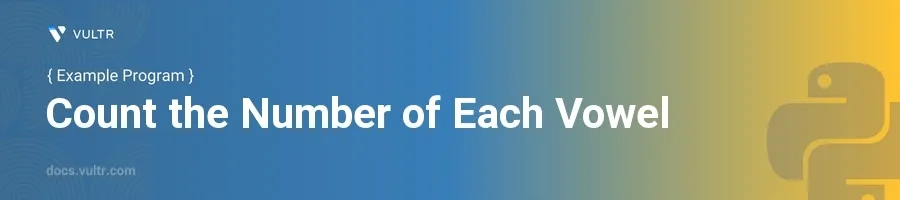
Introduction
Counting vowels in a string is a common problem that can be solved efficiently using Python. This operation is fundamental in text processing, data analysis, and natural language processing applications where understanding the composition of texts is crucial.
In this article, you will learn how to count the number of each vowel in a string using Python. Various methods will be demonstrated, ranging from the use of simple loops to more advanced techniques involving dictionaries and list comprehensions. Each method will be illustrated with clear examples to ensure you can apply these solutions in your own projects.
Using a Simple Loop
Count Each Vowel Individually
Initialize a counter for each vowel: a, e, i, o, u.
Iterate through each character in the string and increment the relevant counter if the character is a vowel.
pythontext = "Hello, welcome to the world of Python programming!" a_count = e_count = i_count = o_count = u_count = 0 for char in text.lower(): if char == 'a': a_count += 1 elif char == 'e': e_count += 1 elif char == 'i': i_count += 1 elif char == 'o': o_count += 1 elif char == 'u': u_count += 1 print("A:", a_count, "E:", e_count, "I:", i_count, "O:", o_count, "U:", u_count)
This code traverses the string
text
, evaluating each character. If it matches a vowel, the corresponding counter is incremented. Thelower()
method ensures that the function is case-insensitive.
Using Dictionaries
Utilize a Dictionary to Simplify Counting
Initialize a dictionary to store vowel keys and their counts.
Iterate through each character and increment the count in the dictionary if the character is a vowel.
pythontext = "Explore Python with practical examples." vowels = 'aeiou' vowel_count = {vowel: 0 for vowel in vowels} for char in text.lower(): if char in vowel_count: vowel_count[char] += 1 print(vowel_count)
Here,
vowel_count
is a dictionary that maintains a count of each vowel encountered in the string. The dictionary comprehension sets initial counts to zero. The loop increases these counts appropriately when vowels are found.
Using List Comprehensions and the collections.Counter
Count Vowels Using Python's Built-In Tools
Utilize
collections.Counter
from the Python standard library to count characters efficiently.Filter the counts using a list comprehension to include only vowels.
pythonfrom collections import Counter text = "Demonstrations are better than explanations." counter = Counter(text.lower()) vowels = 'aeiou' vowel_count = {vowel: counter[vowel] for vowel in vowels if vowel in counter} print(vowel_count)
Counter
automatically tallies the frequency of each character in the string. The list comprehension then extracts the counts for vowels. This method is very efficient for long strings.
Conclusion
Counting vowels in a string can be handled through multiple techniques in Python, from basic loops to sophisticated dictionary operations and the use of built-in classes like collections.Counter
. The choice of method depends on factors such as code clarity, efficiency, and your specific use case requirements. By mastering these techniques, you ensure your text-processing tools are versatile and robust, ready to handle a variety of challenges in data handling and analysis.
No comments yet.