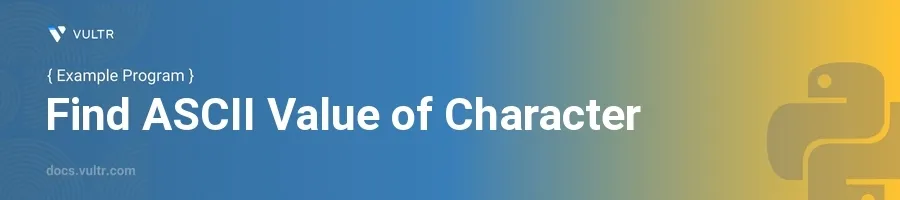
Introduction
The ASCII (American Standard Code for Information Interchange) standard assigns a numerical value to a set of printable characters and a few control characters. Understanding ASCII values is essential for various computing tasks, especially those related to data encoding, processing, and character representation in programming.
In this article, you will learn how to find the ASCII value of characters in Python. You'll explore several examples that illustrate different methods and scenarios where you might need to retrieve these values.
Finding ASCII Value Using ord()
Basic Usage of ord()
Start by choosing a character whose ASCII value you need.
Use Python's built-in
ord()
function to obtain the ASCII value.pythonchar = 'A' ascii_value = ord(char) print(ascii_value)
This code outputs the ASCII value of the character
'A'
, which is 65. Theord()
function takes a string argument of a single Unicode character and returns its ASCII/Unicode ordinal.
ASCII Values in a String
Define a string with multiple characters.
Loop through the string and find the ASCII value of each character using
ord()
.pythontext = 'Hello' ascii_values = [ord(char) for char in text] print(ascii_values)
This script converts each character in the string 'Hello' into its corresponding ASCII value, resulting in the list
[72, 101, 108, 108, 111]
.
Converting Special Characters and Symbols
ASCII for Non-Alphabetic Characters
Include numeric and special symbols in your example.
Apply the
ord()
function to see their ASCII representations.pythonsymbols = '!@3' symbol_values = [ord(symbol) for symbol in symbols] print(symbol_values)
For the string consisting of special characters '!', '@', and the numeral '3', the script returns their ASCII values
[33, 64, 51]
.
Handling Unicode Characters
Use Unicode characters to understand how
ord()
extends beyond standard ASCII.Retrieve the Unicode points for these characters.
pythonunicode_chars = 'áêô' unicode_values = [ord(uch) for uch in unicode_chars] print(unicode_values)
This example demonstrates the ASCII value for extended characters like 'á', 'ê', and 'ô', showing their respective Unicode points
[225, 234, 244]
.
Conclusion
Using Python to find the ASCII value of characters is straightforward with the ord()
function. This capability is particularly useful when you need to perform character manipulations, encoding, or when working with data transmission protocols that depend on ASCII values. By mastering these techniques, enhance your data processing tasks by ensuring you can handle and transform any character data efficiently.
No comments yet.