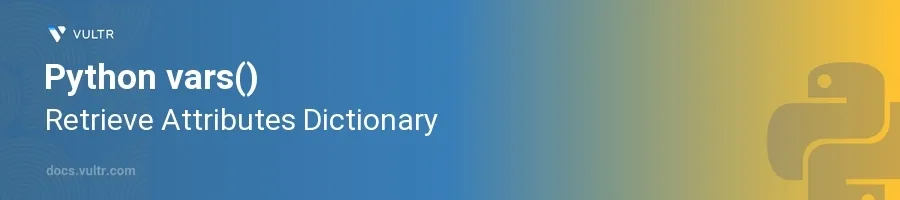
Introduction
The ascii()
function in Python converts any object into a readable ASCII-encoded version, which substitutes any non-ASCII characters with escape characters. This function is especially useful for representing complex data structures or characters that may not be directly displayable or readable in standard output environments, such as logs or ASCII-only systems.
In this article, you will learn how to use the ascii()
function to handle various data types, including strings with special characters, lists, and custom objects. Discover techniques to effectively manage encoding while ensuring the readability of your output in different programming situations.
Using ascii() with Strings
Handle Special Characters in Strings
Create a string containing special or non-ASCII characters.
Apply the
ascii()
function to achieve a safe ASCII representation.pythonspecial_string = 'Café Münch' ascii_representation = ascii(special_string) print(ascii_representation)
Here,
ascii(special_string)
converts non-ASCII characters inspecial_string
to their escaped, ASCII-compatible representations. The output will display escape sequences for characters like 'é' and 'ü'.
Visualize Control Characters in Strings
Include control characters or escape sequences in a string.
Use
ascii()
to visualize these characters explicitly.pythonstring_with_control = "Line1\nLine2\tTab" ascii_output = ascii(string_with_control) print(ascii_output)
This code snippet displays the newline (
\n
) and tab (\t
) as literal characters in their escaped forms, allowing for easier debugging and logging of strings containing such characters.
Using ascii() with Collections
Arrays and Lists
Create a list containing a mix of numeric, string, and special characters.
Convert this list to its ASCII representation.
pythonmixed_list = [1, 'apple', 'naïve', 10.5] ascii_list = ascii(mixed_list) print(ascii_list)
The function
ascii(mixed_list)
converts the entire list into a string while encoding non-ASCII characters in the list items, such as 'ï'.
Dictionaries
Construct a dictionary with ASCII and non-ASCII keys or values.
Render this dictionary in an ASCII-safe version for display or logging.
pythoncustom_dict = {'age': 25, 'name': 'José'} ascii_dict = ascii(custom_dict) print(ascii_dict)
Executing
ascii(custom_dict)
will translate the dictionary into a string form where non-ASCII characters in values (or keys) are safely encoded.
Using ascii() with Custom Objects
Define a Custom Object
Define a class with a
__str__
or__repr__
method that returns a string with special characters.Instantiate the class and use
ascii()
to get an ASCII version of its string representation.pythonclass Fruit: def __init__(self, name): self.name = name def __repr__(self): return f'Fruit(name="{self.name}")' fruit = Fruit("Bañana") print(ascii(fruit))
This snippet will return the string representation of the
fruit
object with non-ASCII characters in its name escaped. This is useful for logging or displaying objects in an ASCII-only context.
Conclusion
The ascii()
function is an invaluable tool in Python for ensuring that data containing non-ASCII characters is properly encoded for environments that require ASCII compatibility. Whether dealing with strings, collections, or custom objects, applying the ascii()
function provides a reliable way to manage character encoding issues, improve code readability, and enhance data logging practices. By mastering the examples provided, streamline the handling of diverse data types in any ASCII-restricted environment.
No comments yet.