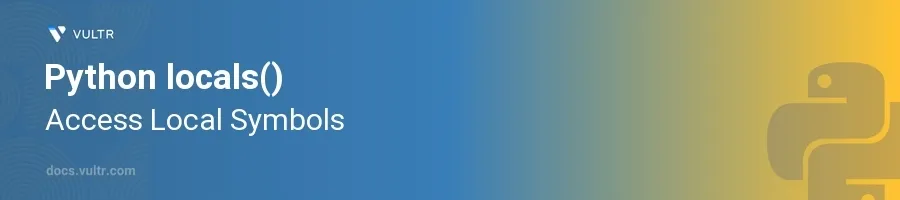
Introduction
The locals()
function in Python provides a way to access the dictionary containing the local symbol table of the current scope, where the function is invoked. It's a built-in function primarily used for debugging purposes, to peek into the values of variables that exist locally in a function or a block scope at any point during execution. This can be particularly helpful for dynamic evaluations and understanding the state of local variables.
In this article, you will learn how to efficiently utilize the locals()
function to access and manipulate local variables in different programming contexts. Explore practical examples that demonstrate its usage and discover some nuances when working with this function.
Accessing Local Variables with locals()
Retrieve Current Local Symbol Table
Define a function to illustrate how
locals()
can be used to capture local variables at runtime.Inside the function, define some local variables and then use
locals()
to display them.pythondef show_locals(): a = 10 b = "Test String" c = [1, 2, 3] local_vars = locals() print(local_vars) show_locals()
This code snippet defines a function
show_locals()
that sets up a few local variables and captures the local symbol table usinglocals()
. The dictionary of local variables is then printed, showing keys as variable names and values as their corresponding values.
Modifying the Local Symbol Table (Caution!)
Understand that modifying the locals dictionary directly is possible, but changes might not reflect in the actual local variables.
Experiment with an example to observe the behavior.
pythondef modify_locals(): x = 10 print("Before:", locals()) locals()['x'] = 20 print("After:", locals()) print("Value of x:", x) modify_locals()
In this example, while the locals dictionary reflects the modification (
x
is changed from 10 to 20), the actual local variablex
remains unchanged. Python’s documentation advises against modifying the locals dictionary, as it may not always work as expected and can lead to unpredictable bugs.
Using locals()
in Loops and Conditionals
Incorporate
locals()
inside a loop to monitor the state of variables dynamically.pythondef loop_locals(): for i in range(3): var_name = 'var_' + str(i) locals()[var_name] = i * 2 print(locals()) loop_locals()
This function,
loop_locals()
, dynamically creates and assigns names to local variables within a loop. It useslocals()
to access and modify the local symbol table. Notice the printed output includes all the generated local variables, showcasing howlocals()
could be used for dynamic assignments and debugging.
Conclusion
The locals()
function in Python serves as a valuable tool for accessing and examining the local symbol table at runtime, enhancing the debugging process and allowing modifications during dynamic evaluations. Despite its ability to manipulate local data, caution is needed due to potential unpredictability. Implement the locals()
function mindfully, primarily for debugging and exploration, to keep your Python scripts robust and maintainable. By leveraging the examples provided, master this tool to enhance your debugging and code introspection skills effectively.
No comments yet.