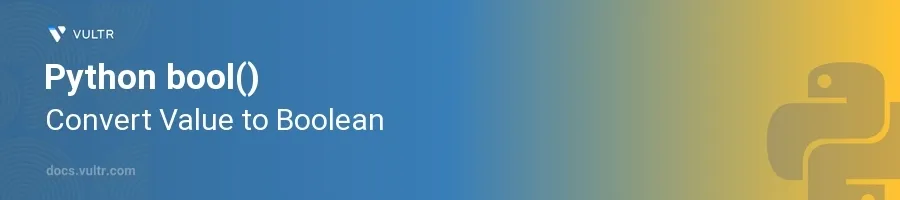
Introduction
The bool()
function in Python is a built-in method used to convert a given value into a Boolean value, either True
or False
. This function is fundamental in Python programming as it helps in making decisions and controlling the flow of execution based on the truthiness or falsiness of different data types and values.
In this article, you will learn how to effectively leverage the bool()
function to assess various data types and values. Explore how different inputs are converted to Boolean values and see practical examples that demonstrate the use of bool()
in real-world programming scenarios.
Understanding the Basic usage of bool()
Determine the Boolean Value of Constants
Use the
bool()
function to check the truthiness of constant values likeNone
,True
,False
.pythonprint(bool(None)) # Outputs: False print(bool(True)) # Outputs: True print(bool(False)) # Outputs: False
Evaluating constants helps identify which are inherently considered true or false in Python.
Convert Numeric Types to Boolean
Apply
bool()
on various numeric types including integers and floats.pythonprint(bool(0)) # Outputs: False print(bool(1)) # Outputs: True print(bool(-1)) # Outputs: True print(bool(0.01)) # Outputs: True print(bool(0.0)) # Outputs: False
Here, any number other than zero is
True
, and zero isFalse
.
Using bool()
with Collections
Evaluate Collections Like Lists, Tuples, and Strings
Test the truthiness of different collections: lists, tuples, and strings.
pythonprint(bool([])) # Outputs: False print(bool([0, 1, 2])) # Outputs: True print(bool("")) # Outputs: False print(bool("Python")) # Outputs: True print(bool(())) # Outputs: False print(bool((1, 2))) # Outputs: True
Collections are
False
if they are empty andTrue
otherwise.
Use bool()
with Dictionaries and Sets
Analyze dictionaries and sets via the
bool()
function.pythonprint(bool({})) # Outputs: False print(bool({'key': 'value'})) # Outputs: True print(bool(set())) # Outputs: False print(bool({1, 2, 3})) # Outputs: True
Like other collections, dictionaries and sets yield
True
if not empty andFalse
if empty.
Practical Applications of bool()
Decision Making and Conditional Statements
Integrate
bool()
in conditional statements for decision making.pythonitems = [1, 2, 3] if bool(items): print("List is not empty") else: print("List is empty")
Determine the presence of items in a list to control the flow of execution.
Filtering Data
Use
bool()
function to filter non-truthy elements from a list.pythondata = [0, False, 'Python', '', 23, []] filtered_data = filter(bool, data) print(list(filtered_data)) # Outputs: ['Python', 23]
This uses
bool()
to remove elements that areFalse
under Boolean evaluation.
Conclusion
Mastering the bool()
function in Python helps in making code more flexible and intuitive in terms of conditional checks and data evaluation. From simple value checking to the more complex filtering of data collections, bool()
proves to be an invaluable tool across numerous scenarios. By integrating these methods into your programs, you ensure that they handle various inputs accurately in conditions and loops, enhancing the robustness and reliability of your code.
No comments yet.