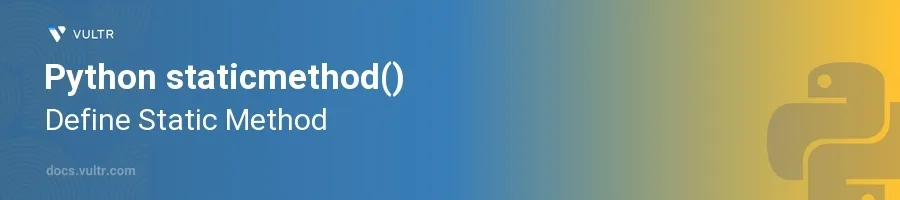
Introduction
The staticmethod()
function in Python is a built-in function that transforms a method into a static method. This means that the method, once defined as static, is not dependent on the class instance. Static methods can be called on the class itself, rather than on instances of the class. They are typically used to create utility functions that are relevant to classes but that do not require a class or instance as a parameter.
In this article, you will learn how to use the staticmethod()
function effectively. Explore scenarios where static methods are particularly useful and see examples of how to implement static methods in your classes.
Understanding Static Methods
Definition and Usage
Recognize that static methods do not access or modify class state.
Understand that they are bound to the class and not the instance of the class.
Note that they can be called using the class name, rather than a class instance.
pythonclass Mathematics: @staticmethod def add(x, y): return x + y # Calling static method result = Mathematics.add(5, 3) print(result)
In this example,
add()
is a static method that performs an addition operation. It is called using the class nameMathematics
and does not require an instance to execute. The output will be8
.
Benefits of Using Static Methods
They help organize utility functions inside a class.
They enhance code readability and maintainability.
Static methods reduce memory usage as they do not require instance creation.
Comparing Static Methods with Class Methods and Instance Methods
Static methods (
@staticmethod
): Use neither the class (cls
) nor the instance (self
) to perform their function.Class methods (
@classmethod
): Use the class (cls
) to execute and can modify the class state.Instance methods: Access and modify the instance (
self
) and class data.
Practical Applications of Static Methods
Scenario: Calculations and Algorithms
Implement mathematical or logical operations that do not depend on class instances.
Use static methods for operations like factorial calculations, Fibonacci series generation, etc.
pythonclass Algorithms: @staticmethod def fibonacci(n): a, b = 0, 1 for _ in range(n): a, b = b, a + b return a print(Algorithms.fibonacci(10))
This code defines a static method
fibonacci()
that generates the nth Fibonacci number. It is a utility method that does not interact with instance or class data.
Scenario: Configuration Data Conversion
Convert or parse configuration data applicable across all instances.
Using static methods for such operations ensures that the method can be accessed universally without instantiation.
pythonclass ConfigParser: @staticmethod def parse(config_str): config_dict = dict(item.split("=") for item in config_str.split(";")) return config_dict config = "path=/usr/bin; max_size=1000" parsed_config = ConfigParser.parse(config) print(parsed_config)
Here, the
parse()
static method is used to convert a configuration string into a dictionary. This utility does not require any specific class or instance attributes.
Conclusion
The staticmethod()
function in Python plays a crucial role in defining methods that do not need an instance for their operations. By learning to use static methods appropriately, you ensure that your class designs are efficient and logically structured. Static methods are best utilized for generic operations applicable across all instances, or when a method operation does not involve the class or instance state. This utility aids in keeping your code organized and directly accessible, promoting cleaner and more pragmatic programming practices.
No comments yet.