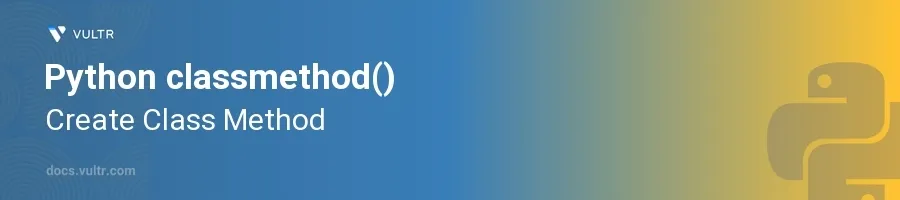
Introduction
The classmethod()
decorator in Python is a built-in function that transforms a method into a class method. Unlike standard methods, class methods are not bound to an instance of the class, but rather to the class itself. This means they can be called on the class directly, not just on an instance. Such methods are particularly useful when you need to perform tasks that are relevant to the entire class instead of individual objects.
In this article, you will learn how to define and use class methods efficiently in your Python code. Explore scenarios where class methods provide advantages over instance methods, such as factory methods and modifying class state.
Understanding classmethod()
Basic Usage of classmethod()
Define a class method using the
@classmethod
decorator.Use the first argument in the method, typically named
cls
, to reference the class.pythonclass Person: species = "Homo sapiens" @classmethod def show_species(cls): print("Species:", cls.species) Person.show_species()
This snippet defines a class
Person
with a class methodshow_species()
that prints the species of the person. When callingshow_species()
on the class, it prints "Species: Homo sapiens".
Modifying Class State
Use a class method to alter class-level attributes, which is useful for updating data shared among all instances.
See the effects on all instances when modifying class attributes via class methods.
pythonclass Counter: count = 0 @classmethod def increment(cls): cls.count += 1 print("Count:", cls.count) Counter.increment() Counter.increment()
Each call to
increment()
increases thecount
class variable by one and prints it. Sincecount
is a class-level attribute, its value is shared across all instances of the class.
Using classmethod() as a Factory
Implement class methods as alternative constructors, commonly known as factory methods.
Use these methods to create class instances using different parameters than those provided by the standard constructor.
pythonclass Book: def __init__(self, title, author): self.title = title self.author = author @classmethod def from_string(cls, data_str): title, author = data_str.split(" - ") return cls(title, author) book = Book.from_string("1984 - George Orwell") print(book.title) # Output: 1984 print(book.author) # Output: George Orwell
The method
from_string()
is a class method that allows creating aBook
instance from a string. This example illustrates the flexibility of class methods for creating instances with different kinds of input data.
Conclusion
Class methods in Python serve pivotal roles when working with class-wide data or when alternative instance creation processes are required. By using the classmethod()
decorator, you enable functions that not only operate on the class itself but also offer utilitarian features such as modifying class properties or serving as factory methods for instance creation. Utilize class methods to maintain clean, organized, and efficient code, especially in complex object-oriented programming scenarios.
No comments yet.