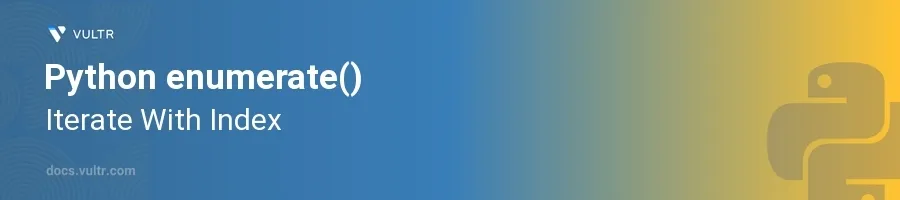
Introduction
The enumerate()
function in Python adds a counter to an iterable and returns it in a form of an enumerate object. This built-in function is particularly useful when you need both the index and the value from a sequence as you iterate through it, making loops clearer and more concise.
In this article, you will learn how to effectively use the enumerate()
function in various programming scenarios. Discover how this function can enhance the readability and efficiency of your code when working with iterables like lists, tuples, and strings.
Using enumerate() with Lists
Iterate Through a List with Indices
Create a list of items.
Use the
enumerate()
function to iterate through the list, obtaining both index and value.pythonfruits = ['apple', 'banana', 'cherry'] for index, fruit in enumerate(fruits): print(f"{index}: {fruit}")
This code prints each fruit in the list along with its corresponding index, providing a clear way to track the position of each item.
Modify List Items Using Indices
Start with an initial list of numerical values.
Use
enumerate()
to iterate over the list and apply modifications based on the index.pythonnumbers = [10, 20, 30, 40] for index, number in enumerate(numbers): numbers[index] = number + 5 print(numbers)
In this snippet, each number in the list
numbers
is increased by 5. Theenumerate()
function helps in accessing the index required for modifying the list in place.
Using enumerate() with Strings
Access Each Character with Its Index
Define a string.
Iterate through the string using
enumerate()
to get each character along with its index.pythontext = "hello" for index, char in enumerate(text): print(f"Character {char} at position {index}")
This example demonstrates how to print each character of the string "hello" with its corresponding index, facilitating operations that may depend on the character positions.
Using enumerate() with Tuples
Enumerate Over a Tuple
Prepare a tuple containing multiple elements.
Use
enumerate()
to loop through the tuple while accessing both the index and the elements.pythontuple_data = (100, 200, 300) for index, value in enumerate(tuple_data): print(f"Index {index} has value {value}")
Here,
enumerate()
is used to print the index and value of each element in the tuple, which is crucial for tasks that require index-based processing.
Conclusion
The enumerate()
function in Python is an invaluable tool for iterating with indices over any iterable object like lists, strings, and tuples. By presenting a simple, clear way to access both the index and the value simultaneously, enumerate()
greatly simplifies code that involves element manipulation based on their positions. Utilize this function in your projects to maintain cleaner and more efficient code, especially in scenarios where detailed tracking of element positions is necessary.
No comments yet.