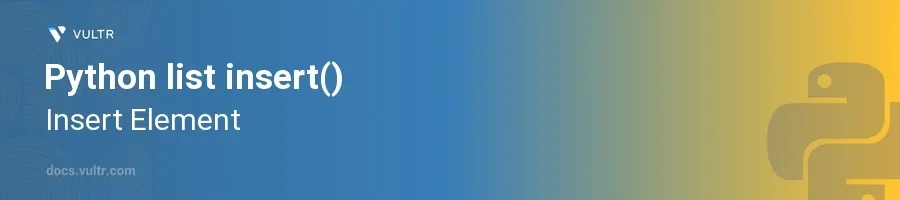
Introduction
The insert()
method in Python provides a way to add elements to a list at a specific index. This method is particularly useful when the order of elements in a list is important and you need to maintain control over where new elements appear. Unlike the append()
method which adds elements only to the end of the list, insert()
offers flexibility in positioning.
In this article, you will learn how to use the insert()
method to add elements to a list at different positions. Explore practical examples that deal with various data types and scenarios to understand how this method operates and the impact it has on the list structure.
Using insert() in Different Scenarios
Insert an Element at the Start of a List
Start with an existing list of elements.
Use the
insert()
method by specifying0
as the index, since list indices start at0
.pythonfruits = ['apple', 'banana', 'cherry'] fruits.insert(0, 'orange') print(fruits)
The list
fruits
initially contains three items. By inserting 'orange' at index 0, it becomes the first item in the list.
Insert an Element at Any Specific Position
Determine the index at which the new element should appear.
Use the
insert()
method and specify the desired index along with the element to insert.pythonnumbers = [1, 2, 3, 5, 6] numbers.insert(3, 4) print(numbers)
Here, the number '4' is inserted at index 3. This places it right between '3' and '5', thereby maintaining the count sequence.
Insert Multiple Elements by Using insert() in a Loop
Plan the positions where elements need to be added.
Use a loop to insert each new element at the appropriate index.
pythonchars = ['a', 'd', 'e'] new_chars = [('b', 1), ('c', 2)] for char, index in new_chars: chars.insert(index, char) print(chars)
This script demonstrates inserting multiple characters into a list to ensure they are in alphabetical order. The tuple
('b', 1)
means insert 'b' at index 1, and similarly, 'c' at index 2.
Handling Edge Cases
Inserting into an Empty List
Always specify index
0
when the list is empty, regardless of what the intended index might be.pythonempty_list = [] empty_list.insert(0, 'first_element') print(empty_list)
The only valid index for an empty list is
0
. Attempting to insert into any other index will have no negative effect but is logically incorrect.
Using a High Index Value
Python allows using an index value greater than the list length, which effectively appends the element to the end.
pythonnumbers = [1, 2, 3] numbers.insert(10, 4) print(numbers)
The number '4' is added to the end of the list because the specified index is beyond the current list size.
Conclusion
The insert()
method in Python enhances the versatility of list manipulations, allowing you to specify exact positions for new elements, which is crucial for maintaining specific sequences or order. These examples illustrate various use cases, from simple insertions to complex manipulations involving multiple elements. Utilize this method to ensure precise control over the structure and content of your lists, making your Python applications more flexible and reliable.
No comments yet.