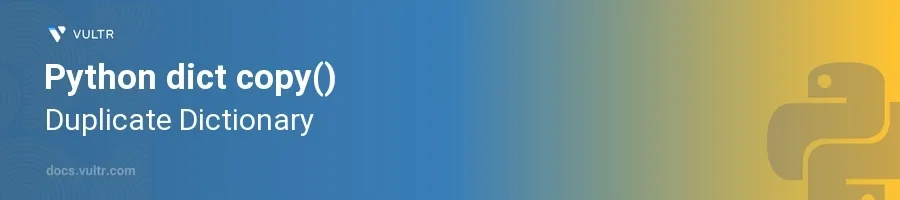
Introduction
The copy()
method in Python is a straightforward way to make a shallow copy of a dictionary. This method is essential when you need to duplicate a dictionary but want to make changes to the new copy without affecting the original dictionary. This technique is often utilized in scenarios where mutable objects, like dictionaries, are manipulated while preserving the base state of the original data.
In this article, you will learn how to use the copy()
method to duplicate dictionaries in Python. Explore how this method works and understand the difference between shallow copying and deep copying, which is particularly important when the dictionaries contain other mutable objects as values.
Basic Usage of copy()
Method
Duplicate a Simple Dictionary
Create a simple dictionary containing key-value pairs.
Use the
copy()
method to duplicate it.pythonoriginal_dict = {'apple': 1, 'banana': 2, 'cherry': 3} copied_dict = original_dict.copy() print(copied_dict)
Here,
copied_dict
becomes a separate dictionary with the same items asoriginal_dict
. Any change made tocopied_dict
does not affectoriginal_dict
.
Modify the Copied Dictionary
Understand that changes to the copied dictionary do not impact the original dictionary.
Modify the copied dictionary and verify the integrity of the original dictionary.
pythoncopied_dict['banana'] = 5 print("Original:", original_dict) print("Copied:", copied_dict)
After updating the value associated with the key
'banana'
incopied_dict
, theoriginal_dict
remains unchanged, confirming the independence of the two dictionaries.
Understanding Shallow Copying
Shallow Copy with Mutable Values
Recognize that a shallow copy duplicates the dictionary’s keys and values, but references to mutable objects in values are the same.
Create a dictionary where values contain mutable objects like lists.
Make a shallow copy and alter the mutable objects.
pythondict_with_list = {'fruits': ['apple', 'banana'], 'vegetables': ['carrot', 'broccoli']} dict_copy = dict_with_list.copy() dict_copy['fruits'].append('cherry') print("Original:", dict_with_list) print("Copied:", dict_copy)
Modifying the list within
dict_copy
affects the same list indict_with_list
since the copy is shallow. Both dictionaries show the updated list, demonstrating that the list object is shared.
Conclusion
The copy()
method in Python provides a convenient way to create a shallow copy of a dictionary. It is ideal for duplicating dictionaries when the values are immutable or when changes to the values will not impact the original dictionary. However, with mutable objects, always consider whether a shallow copy meets the needs, or if a deep copy (using copy.deepcopy
) is necessary to completely isolate the duplicate from the original. By understanding and implementing the concepts shared, you ensure your dictionary manipulations are effective and error-free.
No comments yet.