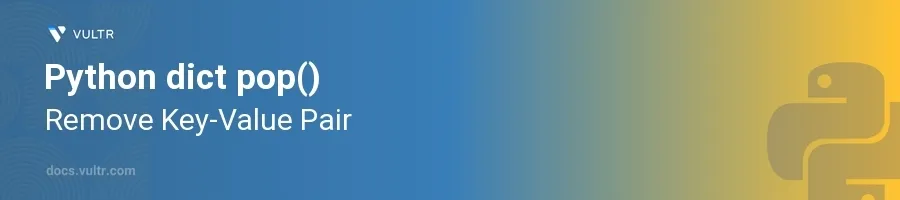
Introduction
The pop()
method in Python dictionaries is an essential tool for managing key-value pairs. It allows for the removal of a specified key from a dictionary and returns the value associated with that key. This functionality is particularly useful in scenarios where dynamic manipulation of data stored in dictionaries is required, such as when processing or filtering data.
In this article, you will learn how to use the pop()
method effectively to remove key-value pairs from dictionaries. You'll explore practical examples to understand better how pop()
works and how it can be utilized in various programming contexts.
Utilizing pop() to Remove Items
Basic Usage of pop()
Start with a dictionary containing some key-value pairs.
Choose a key to remove and use the
pop()
method.pythonperson = {'name': 'John', 'age': 30, 'city': 'New York'} age = person.pop('age') print(person) print("Removed age:", age)
This example removes the key
'age'
from theperson
dictionary and stores the removed value in the variableage
. The updated dictionary no longer contains the'age'
key.
Handling Non-existent Keys
Prepare for situations where the key might not exist by using the optional default argument in
pop()
.Attempt to remove a non-existent key with a default value to avoid
KeyError
.pythonperson = {'name': 'Alice', 'city': 'Los Angeles'} age = person.pop('age', None) print("Age:", age)
In this case, since
'age'
does not exist in theperson
dictionary,pop()
returnsNone
instead of throwing aKeyError
. The dictionary remains unchanged.
Using pop() in Conditional Statements
Apply
pop()
within if-else structures to dynamically manage dictionary contents based on certain conditions.pythondata = {'key1': 100, 'key2': 200, 'key3': 300} if 'key2' in data: value = data.pop('key2') print("Removed key2 with value:", value) else: print("Key not found!")
This snippet checks if
'key2'
exists in the dictionary before attempting to pop it. It safely removes the key-value pair if the key exists.
Conclusion
The pop()
function in Python dictionaries is a versatile method for safely managing and manipulating key-value pairs. It not only removes the key-value pair but also provides options for handling cases where keys do not exist, thereby preventing runtime errors. By incorporating pop()
into your Python coding practices, you ensure that your dictionary manipulations are efficient and error-free. Experiment with these techniques to maintain cleaner and more dynamic code when working with dictionaries.
No comments yet.