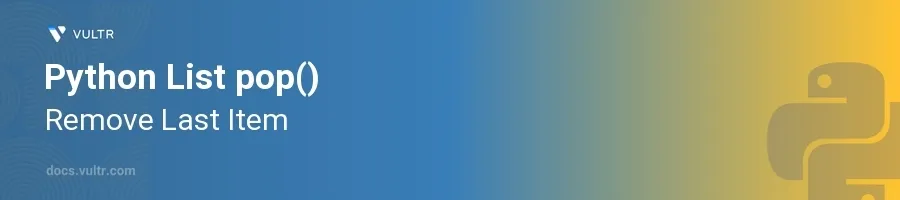
Introduction
The pop()
method in Python is a versatile function used to remove an item from a list. Specifically, it's highly efficient when you need to delete the last item of a list, but it can also be adjusted to remove an item from any position. The pop()
method not only deletes the element but also returns it, making it possible to capture the removed item if needed.
In this article, you will learn how to use the pop()
method effectively to manage list items. Explore various scenarios where pop()
can be employed to manipulate list data dynamically, from basic removal of the last item to more complex usages involving conditional removals.
Basic Usage of pop()
Remove the Last Item from a List
Ensure you have a non-empty list.
Use the
pop()
method to remove the last item.pythonfruits = ['apple', 'banana', 'cherry'] last_fruit = fruits.pop() print(last_fruit) print(fruits)
This example demonstrates removing the last item, 'cherry', from the
fruits
list. After popping, 'cherry' is captured inlast_fruit
, and the list updates to reflect the removal.
Remove an Item at a Specific Index
Specify the index of the item to remove.
Apply the
pop()
method with the index as an argument.pythonfruits = ['apple', 'banana', 'cherry'] removed_fruit = fruits.pop(1) print(removed_fruit) print(fruits)
In the above code,
pop(1)
removes 'banana' from the specified index. The function returns 'banana', which is stored inremoved_fruit
, and updates the list accordingly.
Handling Exceptions with pop()
Safely Using pop() with Error Handling
Anticipate the possibility of an empty list or an invalid index.
Enclose the
pop()
operation within a try-except block to catch exceptions and handle them gracefully.pythonfruits = [] try: fruit = fruits.pop() print(fruit) except IndexError as e: print("Error:", str(e))
This code snippet checks if the list is empty before attempting to pop an item. If the list is empty (
fruits
),pop()
raises anIndexError
, which is caught and handled by printing an error message.
Conclusion
The pop()
function in Python serves as a powerful tool for dynamically managing lists by both removing and returning elements. Mastery of pop()
enhances your ability to manipulate lists, capable of removing items by index and handling potential errors effectively. Apply these techniques to ensure your list manipulations are both efficient and robust, keeping your code clean and functional.
No comments yet.