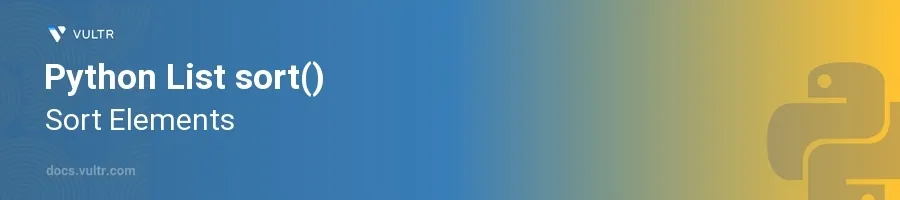
Introduction
The sort()
method in Python provides a simple and efficient way to order the elements within a list. Whether you need to sort items numerically or alphabetically, this method adjusts the list in place, making it a preferred option for modifying lists directly without creating a new sorted list.
In this article, you will learn how to use the sort()
method effectively in various scenarios. Discover the straightforward and advanced capabilities of this function as you explore its parameters like key functions and sorting order.
Sorting Basic Lists
Sort a List in Ascending Order
Create a list of numbers or strings.
Call the
sort()
method on the list.pythonitems = [10, 1, 7, 4, 3, 9, 2] items.sort() print(items)
This code sorts the list
items
in ascending order. The list is modified in place and now appears in sorted form.
Sort a List in Descending Order
Specify the
reverse
parameter asTrue
to sort the list in descending order.Apply the
sort()
method on your list.pythonitems = [10, 1, 7, 4, 3, 9, 2] items.sort(reverse=True) print(items)
Using this method,
items
now displays in descending order.
Advanced Sorting Techniques
Sorting with Custom Key Functions
Utilize the
key
parameter to define a custom sort basis.Provide a function that processes elements before they're compared.
python# Sorting strings by length words = ['banana', 'apple', 'cherry', 'date'] words.sort(key=len) print(words)
Here, the list
words
is sorted by the length of each string becauselen
is passed to thekey
parameter.
Sorting Complex Structures
For items like lists of tuples or dictionaries, leverage a lambda function as the key.
Define precisely which element to consider during sorting.
python# Sorting a list of tuples by the second element tuples = [(1, 'one'), (3, 'three'), (4, 'four'), (2, 'two')] tuples.sort(key=lambda x: x[1]) print(tuples)
This block sorts
tuples
based on each tuple's second element (the string), ordering them alphabetically.
Conclusion
The sort()
method is a versatile tool in Python for modifying lists directly and efficiently. With its ability to sort in ascending or descending order and the option to use custom key functions, sort()
handles both simple and complex data structures effectively. Employ this method in your Python projects to organize data seamlessly and increase the clarity and efficiency of your code.
No comments yet.