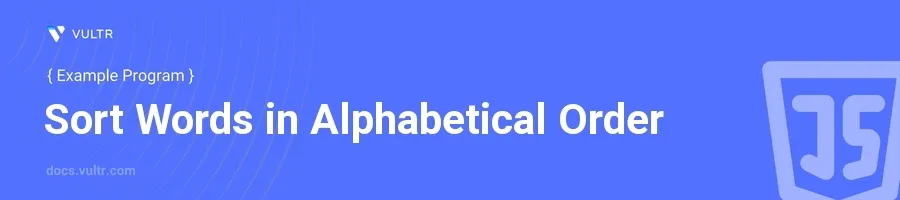
Introduction
Sorting words in alphabetical order is a common task in many programming scenarios, from organizing user input to managing data for display. JavaScript, with its flexible string manipulation capabilities, provides several methods to accomplish this task efficiently.
In this article, you will learn how to sort words in alphabetical order using JavaScript. Explore practical examples that demonstrate sorting simple word lists as well as handling strings with multiple words.
Sorting Simple Word Arrays
When dealing with an array of words, JavaScript's Array.prototype.sort()
method offers a straightforward way to arrange words alphabetically. Here’s how to use it:
Define an array containing the words you want to sort.
Apply the
sort()
method to this array.javascriptlet words = ['Banana', 'Apple', 'Orange']; words.sort(); console.log(words);
This code snippet sorts the array
words
alphabetically. Thesort()
method, by default, converts elements to strings and compares their sequences of UTF-16 code unit values.
Sorting Strings with Multiple Words
To sort a string containing multiple words, follow these steps:
Convert the string into an array using the
split()
method.Sort the array using
sort()
.Join the array back into a single string with
join()
.javascriptlet sentence = "zebra apple moon ball"; let sortedSentence = sentence.split(' ').sort().join(' '); console.log(sortedSentence);
In this example, the string
sentence
is first split into an array of words. Thesort()
method sorts these words alphabetically, andjoin(' ')
concatenates them back into a single string with spaces in between.
Handling Case Sensitivity During Sort
JavaScript sorting can be case-sensitive, which might not be desirable. To perform a case-insensitive sort, modify the sort comparison like this:
Apply the
sort()
method with a custom comparator function that compares lowercased versions of words.javascriptlet words = ['banana', 'Apple', 'orange']; words.sort((a, b) => a.toLowerCase().localeCompare(b.toLowerCase())); console.log(words);
This code snippet includes
toLowerCase()
to normalize the case of the words before comparison, ensuring that the order is strictly alphabetical regardless of the original word casing.
Conclusion
Sorting words in alphabetical order in JavaScript is straightforward with the sort()
method. Whether you're working with simple arrays or strings of text, JavaScript provides efficient techniques to order your data alphabetically. Using basic array manipulations like split()
, sort()
, and join()
, as well as employing custom sort functions to handle case sensitivity, you can effectively organize words in the order you need. Implement these strategies to enhance data readability and management in your JavaScript applications.
No comments yet.