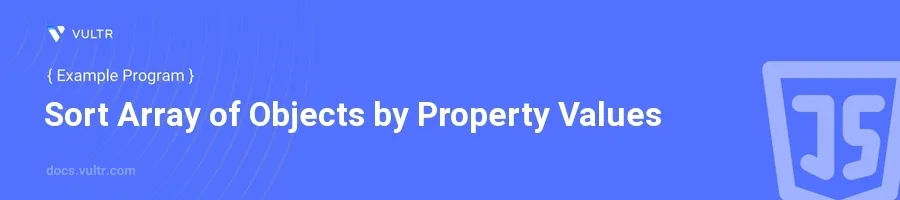
Introduction
Sorting an array of objects by property values is a common task in JavaScript programming. This requires understanding how to leverage the sort()
method, which is a versatile method in JavaScript's array prototype. This method allows you to order the elements of an array based on some condition set by a callback function.
In this article, you will learn how to effectively sort an array of objects by different property values using a few examples. You'll discover how to sort based on numerical values, string values, and even on multiple properties. Each example will guide you through the process and explain the underlying logic, ensuring you can apply these methods to your specific needs.
Sorting by Numeric Properties
Sort Objects by a Numeric Property
Define an array of objects where each object has at least one numeric property.
Use the
sort()
method with a custom comparator function to order the array based on this numeric property.javascriptconst users = [ { name: "Alice", age: 25 }, { name: "Bob", age: 20 }, { name: "Claire", age: 30 } ]; users.sort((a, b) => a.age - b.age); console.log(users);
This code sorts the users array by the age property in ascending order. The comparator function takes two arguments (two elements from the array) and returns a difference between their age properties.
Sort Objects in Descending Order
Modify the comparator function to sort the array in descending order based on a numeric property.
javascriptusers.sort((a, b) => b.age - a.age); console.log(users);
By swapping
a
andb
in the subtraction, the array is sorted in descending order. The youngest person comes last in this sorted array.
Sorting by String Properties
Sort Objects by a String Property
Utilize the
localeCompare()
method in your comparator function to sort objects by a string property.javascriptconst products = [ { name: "Keyboard", price: 30 }, { name: "Mouse", price: 20 }, { name: "Monitor", price: 300 } ]; products.sort((a, b) => a.name.localeCompare(b.name)); console.log(products);
In this example, products are sorted alphabetically by the product name.
localeCompare()
is used because it provides a consistent way to handle string comparison across different locales and languages.
Case Insensitive Sorting
Adjust the sorting method to handle case-insensitive comparisons.
javascriptproducts.sort((a, b) => a.name.toLowerCase().localeCompare(b.name.toLowerCase())); console.log(products);
This modification ensures the sorting ignores case differences by converting strings to lower case before comparing them.
Sorting by Multiple Properties
Combining Numeric and String Sorting
Combine conditions in your sorting logic to sort by multiple properties.
javascriptconst library = [ { title: "The Road Ahead", author: "Bill Gates", year: 1995 }, { title: "Walter Isaacson", author: "Steve Jobs", year: 2011 }, { title: "Mockingjay", author: "Suzanne Collins", year: 2010 } ]; library.sort((a, b) => { return a.year - b.year || a.title.localeCompare(b.title); }); console.log(library);
This code first sorts the books by
year
. If the year is the same, thenlocaleCompare()
is used to sort bytitle
. This ensures a stable and multi-level sorting mechanism, useful for more complex data structures.
Conclusion
Sorting arrays of objects by property values in JavaScript is a straightforward process once you master the use of the sort()
method with appropriate comparator functions. From numeric and string properties to more complex multi-property sorting, the flexibility of sort()
allows it to meet a wide range of sorting needs. Adapt the examples provided to fit the specific structure of your data and ensure your arrays are always well-organized according to your application's requirements. By mastering these techniques, you enhance data handling and manipulation capabilities in your JavaScript projects.
No comments yet.