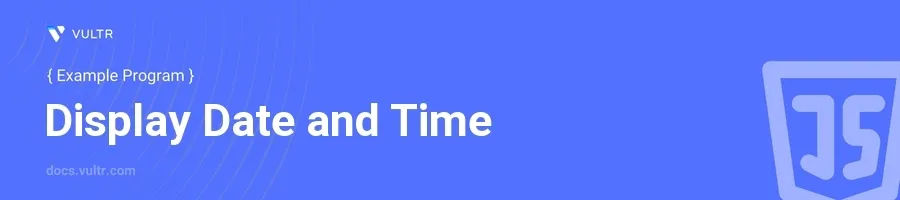
Introduction
Displaying the date and time is a fundamental task in many web applications, from blogs showing post dates to e-commerce sites tracking order times. JavaScript, being a core technology of the web, provides robust methods to handle date and time efficiently. Understanding how to manipulate these values can enhance user experience by providing timely information updates.
In this article, you will learn how to effectively display the date and time using JavaScript. Discover various methods to get the current date and time, format it according to different requirements, and update it dynamically on a web page.
Retrieving Current Date and Time
Display the Complete Date and Time
Generate a new Date object to get the current date and time.
Convert the Date object to a string for easy reading.
javascriptconst now = new Date(); console.log(now.toString());
This code creates a new
Date
object and assigns it to the variablenow
. ThetoString
method converts this date into a readable string format.
Displaying Only the Date
Access only the date part by using the
toLocaleDateString()
method.javascriptconst today = new Date().toLocaleDateString(); console.log(today);
toLocaleDateString()
formats the date according to the locale of the environment where the script runs, typically rendering it in a familiar format to the user.
Displaying Only the Time
Use the
toLocaleTimeString()
method to extract only the time part from the current date.javascriptconst timeNow = new Date().toLocaleTimeString(); console.log(timeNow);
This method outputs the current time formatted according to the user's locale, focusing exclusively on hours, minutes, and sometimes seconds.
Formatting Date and Time
Custom Date and Time Format
Use options with
toLocaleString()
to specify how date and time should appear.javascriptconst options = { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric', hour: '2-digit', minute: '2-digit', second: '2-digit' }; const customDateTime = new Date().toLocaleString('en-US', options); console.log(customDateTime);
The
options
object here dictates exactly how the date and time should be formatted: including the day of the week, full year, month name, day of the month, and time with seconds.
Dynamically Updating Date and Time on a Web Page
Creating an Auto-updating Clock
Set up an HTML element to display the time.
Use
setInterval
to update this element every second.html<span id="clock"></span>
javascriptfunction showTime() { const timeNow = new Date().toLocaleTimeString(); document.getElementById('clock').textContent = timeNow; } setInterval(showTime, 1000);
The
showTime
function fetches the current time and updates thetextContent
of the HTML element with the idclock
every 1000 milliseconds (or every second). This keeps the displayed time current to the second.
Conclusion
Displaying the date and time in JavaScript is essential for many web applications. Armed with these techniques, integrate real-time date and time functionalities in web-based projects with ease. Adopt Date
object methods like toLocaleDateString()
, toLocaleTimeString()
, and toLocaleString()
to format and control the output based on user locale, custom preferences, and dynamic needs. Leverage JavaScript's capabilities to enhance the interactivity and relevancy of your applications for a better user experience.
No comments yet.