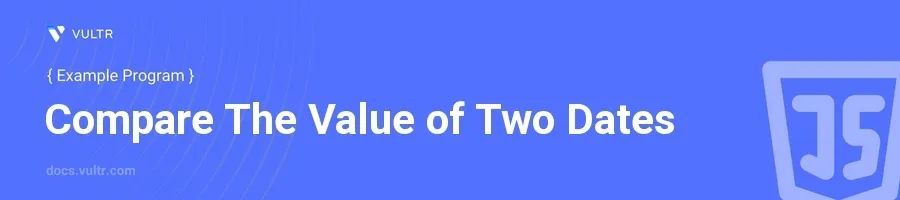
Introduction
Comparing two dates in JavaScript is a common task in web development, particularly in scenarios involving scheduling, calendars, or any application relying on date metrics. Whether determining the time elapsed between two dates, or validating if one date falls before another, handling date comparison is crucial for dynamic and interactive web applications.
In this article, you will learn how to effectively compare the value of two dates using JavaScript. Explore practical examples illustrating how to check if one date is greater than, less than, or equal to another date, and how to find the difference between two dates.
Comparing Two Dates Directly
Check if One Date Comes Before or After Another
Instantiate two date objects.
Use the comparison operators to compare these dates directly.
javascriptvar date1 = new Date('2023-01-01'); var date2 = new Date('2023-01-02'); console.log(date1 < date2); // true console.log(date1 > date2); // false console.log(date1 === date2); // false (different objects in memory)
This example creates two date objects and compares them using
<
,>
and===
. Since dates in JavaScript are objects, the===
operator compares memory locations rather than values, resulting infalse
even for the same date values, hence it is not used for date value comparison.
Equality Check Using getTime Method
Convert both date objects to their millisecond representations using
getTime()
.Compare these values for equality.
javascriptvar date1 = new Date('2023-01-01'); var date2 = new Date('2023-01-01'); console.log(date1.getTime() === date2.getTime()); // true
This code snippet accurately checks for equality by converting the date objects to the number of milliseconds since the Unix epoch using
getTime()
, ensuring a correct value comparison.
Finding the Difference Between Two Dates
Calculate the Difference in Days
Instantiate the two date objects.
Get the time value of each date in milliseconds.
Subtract one time value from the other to find the difference in milliseconds.
Convert the difference from milliseconds to days.
javascriptvar date1 = new Date('2023-01-10'); var date2 = new Date('2023-01-01'); var difference = date1 - date2; var days = difference / (1000 * 3600 * 24); console.log(days); // 9
In this code, the subtraction results in a difference expressed in milliseconds. By dividing by the number of milliseconds in a day (
1000 * 3600 * 24
), you convert the result to days.
Conclusion
Comparing and manipulating dates in JavaScript can be handled elegantly using native Date objects and their methods. Checking if dates are equal, determining which date precedes, and computing the difference in time are essential for any application involving date manipulations. By integrating these methods, you harness the full potential of JavaScript to manage date-related data effectively. These tips are instrumental for developers looking to handle date-time operations efficiently in their projects.
No comments yet.