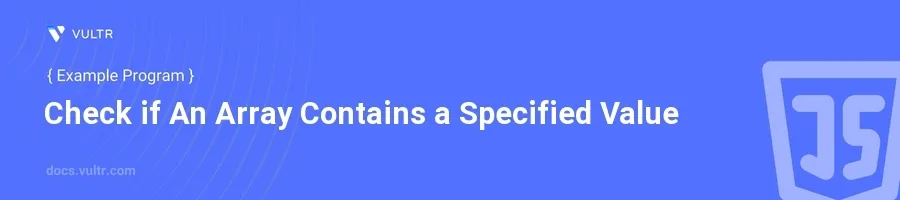
Introduction
JavaScript arrays are versatile structures used for storing multiple values in a single variable. One common task when working with arrays is checking if they contain a specific element. This operation is crucial in many web development scenarios, such as form validation, feature toggling, or conditional rendering based on user input or preferences.
In this article, you will learn how to check if an array contains a specified value in JavaScript. Explore several methods, including using the includes()
method, the indexOf()
method, and leveraging the some()
method for more complex conditions. Each method will be demonstrated with examples to ensure you can implement them effectively in your own projects.
Basic Check with includes()
Direct Value Checking
Start with the simplest and most direct method using
includes()
to check for a value.Create an array and use
includes()
to determine if a specified value is present.javascriptconst fruits = ['apple', 'banana', 'cherry']; const hasBanana = fruits.includes('banana'); console.log(hasBanana); // Output: true
This example demonstrates checking for 'banana' in the
fruits
array. Theincludes()
method returnstrue
since 'banana' is indeed in the array.
Finding Value with indexOf()
Testing Presence Through Index
Use
indexOf()
when you need to know the index of the element as well.Check if the returned index is not
-1
to confirm presence.javascriptconst pets = ['dog', 'cat', 'parrot']; const index = pets.indexOf('cat'); console.log(index !== -1); // Output: true
The
indexOf()
method returns the first index at which a given element can be found, or-1
if it is not present. Here, since 'cat' is found, the index is 0, and the conditionindex !== -1
evaluates totrue
.
Use some()
for Conditional Checking
Evaluating with Functions
Implement
some()
when the condition for inclusion is more complex than a direct match.Define an array with objects and use a function to check for a condition.
javascriptconst people = [ { name: 'John', age: 25 }, { name: 'Jane', age: 30 } ]; const hasMinor = people.some(person => person.age < 18); console.log(hasMinor); // Output: false
In this example,
some()
checks each object in thepeople
array to see if any individual is a minor (under 18 years old). It returnsfalse
because all listed individuals are 18 or older.
Conclusion
Checking if an array contains a specified value is an essential operation in JavaScript programming. With the methods discussed—includes()
, indexOf()
, and some()
—you can efficiently determine the presence of elements under various conditions. Use includes()
for straightforward presence checks, indexOf()
when the position is also required, and some()
for evaluating more complex conditions. By mastering these techniques, ensure your arrays are processed accurately and your code remains robust and reliable.
No comments yet.