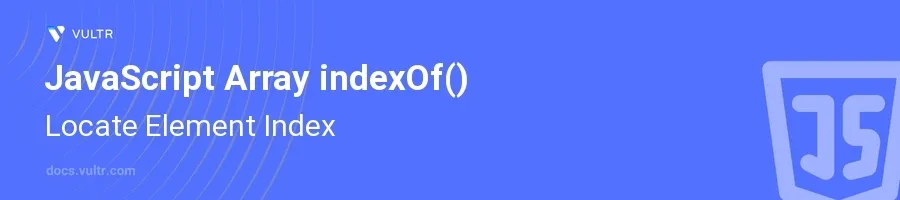
Introduction
The indexOf()
method in JavaScript is an essential tool for working with arrays. It provides a straightforward way to locate the position of a specified element within an array. By returning the first index at which a given element can be found, or -1 if it is not present, this method is pivotal for determining if and where specific data exists in an array.
In this article, you will learn how to effectively utilize the indexOf()
method in various scenarios. Explore practical examples that demonstrate finding the position of elements in arrays and handling cases where the element does not exist.
Basic Usage of indexOf()
Locate an Element in an Array
Define an array with several elements.
Use the
indexOf()
method to find the index of a specific element.javascriptconst fruits = ['apple', 'banana', 'cherry', 'date']; const index = fruits.indexOf('cherry'); console.log(index);
This code snippet searches for the string
'cherry'
in thefruits
array. Since 'cherry' is located at the third position,indexOf()
returns2
(as array indices start from 0).
Handling Elements Not Found
Try to find an element that does not exist in the array.
Check if the result is
-1
to determine element's absence.javascriptconst fruits = ['apple', 'banana', 'cherry', 'date']; const index = fruits.indexOf('mango'); // mango is not in the array console.log(index);
Since 'mango' is not in the array,
indexOf()
returns-1
. This indicates the absence of the element in the array.
Advanced Use of indexOf()
Using indexOf() to Avoid Duplicates
Before adding an element to an array, check if it is already present using
indexOf()
.Add the element only if it is not already included.
javascriptconst numbers = [1, 2, 3, 4, 5]; let numberToAdd = 3; if (numbers.indexOf(numberToAdd) === -1) { numbers.push(numberToAdd); } else { console.log(`${numberToAdd} already exists in the array.`); }
This snippet prevents adding
3
to the array again since it is already present. It promotes effective management of duplicates in data collections.
Finding Multiple Instances
Loop through the array and use
indexOf()
in each iteration to find all occurrences of an element.Start each subsequent
indexOf()
search from the last found index plus one.javascriptconst characters = ['a', 'b', 'a', 'c', 'a', 'b']; const target = 'a'; let index = characters.indexOf(target); while (index !== -1) { console.log(`Found ${target} at index ${index}`); index = characters.indexOf(target, index + 1); }
The loop in this code continues to search for the character 'a' until
indexOf()
eventually returns-1
. Eachconsole.log
call reports the position of 'a' in thecharacters
array.
Conclusion
The indexOf()
function in JavaScript is a straightforward but powerful tool for searching the position of elements within an array. It provides essential functionality for conditionally manipulating arrays based on element existence and position. Through the examples provided, you can effectively use indexOf()
to check for element presence, manage duplicates, and locate multiple occurrences of the same item. Leverage these strategies to enhance array handling in your JavaScript projects.
No comments yet.