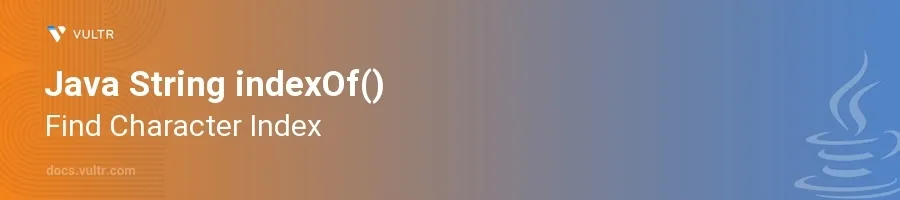
Introduction
The indexOf()
method in Java is a powerful tool used to find the position of a specific character or substring within a string. This function returns the index within the calling String object of the first occurrence of the specified value, starting the search at fromIndex
. If the value is not found, the method returns -1. This functionality makes indexOf()
invaluable for string manipulation tasks, such as parsing and data validation.
In this article, you will learn how to effectively use the indexOf()
method in various scenarios. Explore how this method can help you determine the presence and position of characters or substrings in strings, and see how it aids in data processing tasks by discovering practical implementation examples.
Understanding the indexOf() Method
Basic Usage of indexOf() to Find a Character
Start with a simple string.
Use
indexOf()
to find the position of a character.javaString example = "hello"; int index = example.indexOf('e'); System.out.println("The index of 'e' is: " + index);
This code finds the index of the character 'e' in the string "hello". Since 'e' is the second character,
indexOf()
returns 1.
Finding a Substring Within a String
Define a string that contains a more complex phrase.
Apply
indexOf()
to find a substring.javaString phrase = "Java is fun"; int substrIndex = phrase.indexOf("fun"); System.out.println("The index of 'fun' is: " + substrIndex);
Here,
indexOf()
checks for the substring "fun". It finds "fun" starting at index 8.
Handling Cases Where the Character Does Not Exist
Use a string where the target character or substring does not exist.
Observe the result of
indexOf()
in such cases.javaString sentence = "This is a test"; int notFoundIndex = sentence.indexOf('x'); System.out.println("The index of 'x' is: " + notFoundIndex);
In this example, since 'x' does not appear in the sentence "This is a test",
indexOf()
returns -1, indicating the absence of the character.
Advanced Uses of indexOf()
Searching From a Specified Index
Sometimes, you might need to start your search from a specific position in the string.
Use the overloaded version of
indexOf()
that accepts a starting index.javaString fullText = "Java and JavaScript"; int indexFrom = fullText.indexOf("Java", 5); System.out.println("The index of 'Java' starting from index 5 is: " + indexFrom);
This snippet demonstrates searching for "Java" starting from index 5. The second "Java" in "JavaScript" begins at index 9, which is found by this method.
Using indexOf() in a Loop for Multiple Occurrences
When you need to find all occurrences of a character or substring, use
indexOf()
in a loop.Adjust the starting index on each iteration.
javaString repeatingStr = "Java, Scala, Java, Kotlin"; int lastIndex = 0; while (lastIndex != -1) { lastIndex = repeatingStr.indexOf("Java", lastIndex); if (lastIndex != -1) { System.out.println("Found 'Java' at index: " + lastIndex); lastIndex += "Java".length(); } }
This code continually searches for "Java" within the string, updating
lastIndex
each time "Java" is found to search beyond the current match. It effectively prints all starting positions of "Java" in the string.
Conclusion
The indexOf()
function in Java is a versatile tool that helps you detect the position of characters or substrings within strings. Whether you need to validate input, parse data, or simply check for the presence of particular characters, indexOf()
efficiently gets the job done. The examples and techniques outlined serve as a foundation for extensive string manipulation tasks, ensuring your code is both efficient and easy to read. Harness the capabilities of 'indexOf()' to enhance your Java programs by implementing robust search operations.
No comments yet.