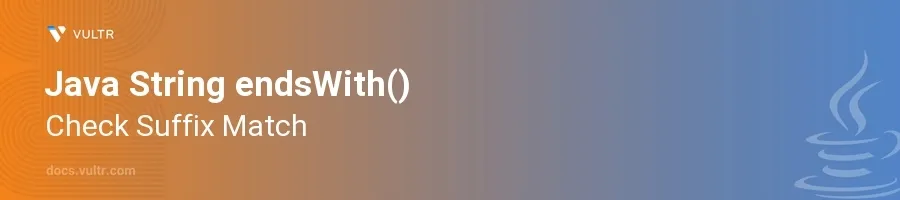
Introduction
The endsWith()
method in Java's String
class is crucial for determining whether a given string ends with a specified suffix. This method simplifies tasks ranging from validation checks to parsing file paths or URLs. Understanding how to effectively use endsWith()
can significantly aid in handling strings according to specific criteria.
In this article, you will learn how to use the endsWith()
method to perform string suffix checks. Discover practical examples that illustrate how to implement this method to confirm file extensions, language codes at the end of URLs, or any general suffix in strings.
Understanding the endsWith() Method
Basic Usage of endsWith()
Start with a basic string declaration.
Use
endsWith()
to determine if the string ends with a specific sequence of characters.javaString filename = "example.pdf"; boolean result = filename.endsWith(".pdf"); System.out.println(result);
This snippet verifies whether the string stored in
filename
ends with the suffix ".pdf". Since it does,endsWith()
returnstrue
.
Case Sensitivity in endsWith()
Recognize that
endsWith()
is case-sensitive.Check the behavior with different case scenarios.
javaString document = "report.DOC"; boolean caseResult = document.endsWith(".doc"); System.out.println(caseResult);
Despite ".DOC" being a valid document format, the method returns
false
because ".doc" does not match ".DOC" exactly due to case sensitivity.
Practical Applications of endsWith()
File Extension Validation
Utilize
endsWith()
for validating file extensions, improving the reliability of file handling operations.Set up multiple checks to ensure the file matches expected document formats.
javaString filePath = "picture.jpg"; boolean isValid = filePath.endsWith(".jpg") || filePath.endsWith(".jpeg"); System.out.println("Is valid image file: " + isValid);
Here,
endsWith()
checks if the file path ends with either ".jpg" or ".jpeg", which confirms whether it's an image file format according to common standards.
Multilingual Website Paths
Deploy
endsWith()
to manage URL paths that include language codes.Ensure the path points to the correct localized version of a website.
javaString url = "https://example.com/about-us/es"; boolean isSpanish = url.endsWith("/es"); System.out.println("Spanish version: " + isSpanish);
This application of
endsWith()
determines if the URL points to the Spanish version of the "about-us" page by checking if it ends with "/es".
General Suffix Checks
Apply
endsWith()
to any string to see if it concludes with a specific suffix, useful in diverse programming contexts.Configure checks that can impact flow control depending on suffix presence.
javaString userStatus = "username_inactive"; boolean isActive = !userStatus.endsWith("_inactive"); System.out.println("User active: " + isActive);
The method checks if
userStatus
ends with "_inactive". The negation operator!
is used soisActive
will betrue
ifuserStatus
does not end with "_inactive".
Conclusion
The endsWith()
function in Java is a simple yet powerful method for verifying whether a string terminates with a specified suffix. Utilize this function in a variety of scenarios, from verifying file formats and handling URL routes to managing system states based on string values. By mastering endsWith()
, you harness a straightforward approach for string-based checks that contribute to cleaner, more efficient Java code. Implement this method in your projects to enhance string processing robustness and reliability.
No comments yet.