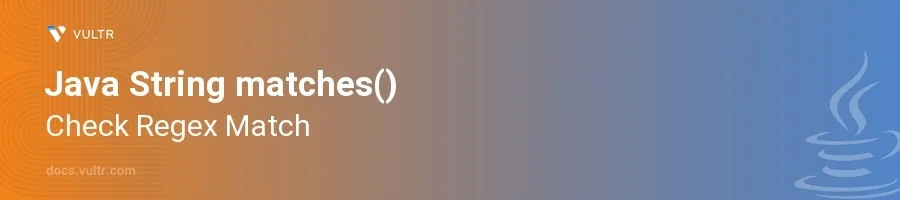
Introduction
The matches()
method in Java is an essential tool for checking if a given string complies with the pattern defined by a regular expression (regex). This method returns a boolean indicating whether the string matches the regex pattern entirely, making it a common choice for validation tasks like format verification and data filtering in Java programming.
In this article, you will learn how to effectively use the matches()
method across various scenarios to validate string patterns. Explore practical examples that demonstrate checking email formats, phone numbers, and more complex patterns to ensure you can apply these techniques to real-world applications.
Understanding the matches() Method
Basic Usage of matches()
Start by defining a string that you want to check.
Specify a regex pattern that defines the rule the string should conform to.
Use the
matches()
method to determine if the string adheres to the pattern.javaString example = "hello"; boolean result = example.matches("hello"); System.out.println(result);
This code snippet evaluates whether the string
example
exactly matches "hello". The output will betrue
as the string perfectly complies with the regex.
Special Characters and Quantifiers in Regex
Learn about special characters like
^
(start),$
(end),.
(any character), and quantifiers like*
(zero or more),+
(one or more) in regex.Formulate a regex pattern using these special characters and quantifiers to perform more dynamic matches.
Apply the
matches()
method with this pattern.javaString phoneNumber = "123-456-7890"; boolean result = phoneNumber.matches("\\d{3}-\\d{3}-\\d{4}"); System.out.println(result);
Here, the regex
\\d{3}-\\d{3}-\\d{4}
checks for a U.S. standard phone number format. The sequence\\d{3}
matches exactly three digits, thus verifying the structure of the phone number.
Practical Regex Match Examples with matches()
Validating Email Addresses
Define a regex pattern for a typical email address.
Create a string that contains an email.
Use
matches()
to confirm if the email string matches the email regex pattern.javaString email = "user@example.com"; boolean isValidEmail = email.matches("^[a-zA-Z0-9_+&*-]+(?:\\.[a-zA-Z0-9_+&*-]+)*@(?:[a-zA-Z0-9-]+\\.)+[a-zA-Z]{2,7}$"); System.out.println("Valid email: " + isValidEmail);
The regex used here includes patterns for the username and the domain, checking for a valid email structure. It ensures the email starts with alphanumeric characters and can contain special characters in specified positions.
Password Complexity Check
Establish a regex that incorporates rules for a strong password (minimum 8 characters, at least one uppercase, one lowercase, and one special character).
Construct a sample password string.
Deploy the
matches()
method to validate the password against the regex pattern.javaString password = "Complex@123"; boolean isStrongPassword = password.matches("^(?=.*[a-z])(?=.*[A-Z])(?=.*\\d)(?=.*[@#$%^&+=]).{8,}$"); System.out.println("Strong password: " + isStrongPassword);
This code checks the strength of a password based on multiple criteria within a single regex expression. Each lookahead (
(?=.*[pattern])
) ensures the presence of at least one lowercase letter, one uppercase letter, one digit, and one special character.
Conclusion
The matches()
function in Java is a highly versatile tool for performing pattern matching and validation using regular expressions. By mastering its usage through the scenarios outlined above, enhance data validation processes with high accuracy and efficiency. Whether checking simple patterns or complex strings, understanding how to craft and utilize regex with the matches()
method advances your Java programming capabilities. Implement these techniques in your projects to ensure robust validation of user input or data processing tasks.
No comments yet.