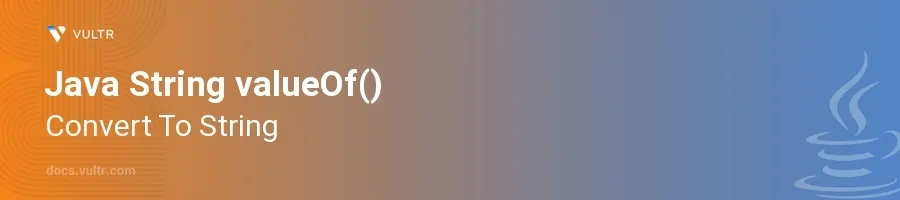
Introduction
The valueOf()
method in Java's String class is a static utility function designed to convert various data types into their string representations. This method proves invaluable when you need to transform primitive data types, objects, or arrays into strings for processing, logging, output display, or further manipulation.
In this article, you will learn how to apply the String.valueOf()
method across different data types including primitives, objects, and arrays. Explore how to harness this method to simplify data representation conversions in Java effectively.
Using String valueOf() with Primitive Data Types
Convert int to String
Define an integer variable.
Use
String.valueOf()
to convert the integer to a string.javaint num = 123; String numStr = String.valueOf(num); System.out.println(numStr);
This code converts the integer
123
to the string "123" and prints it.
Convert boolean to String
Specify a boolean variable.
Convert this boolean to a string using
String.valueOf()
.javaboolean flag = true; String flagStr = String.valueOf(flag); System.out.println(flagStr);
In this snippet, the boolean value
true
is converted to the string "true".
Convert char to String
Initialize a char variable.
Apply
String.valueOf()
to change the char into a string.javachar letter = 'A'; String letterStr = String.valueOf(letter); System.out.println(letterStr);
The character
'A'
is converted and displayed as the string "A".
Using String valueOf() with Objects
Convert Objects to String
Create a simple custom object.
Convert the object to a string using
String.valueOf()
.javaObject obj = new Object(); String objStr = String.valueOf(obj); System.out.println(objStr);
The method converts the object's memory address or hash code (default Object
toString()
method) into a string form.
Apply String valueOf() on Null Object
Declare an object as null.
Safely convert this null object using
String.valueOf()
.javaObject nullObj = null; String nullStr = String.valueOf(nullObj); System.out.println(nullStr); // Prints "null"
This technique ensures no
NullPointerException
is thrown and "null" is returned as a string, showcasing the method's safety in handling null values.
Using String valueOf() with Arrays
Convert Array of Characters to String
Initialize an array of characters.
Convert the entire array into a string using
String.valueOf()
.javachar[] charArray = {'J', 'a', 'v', 'a'}; String arrayStr = String.valueOf(charArray); System.out.println(arrayStr); // Outputs "Java"
This approach transforms an array of characters directly into a string.
Handling Double Arrays
Understand that directly converting non-character arrays like double[] doesn't yield the actual contents:
Demonstrate transforming a double array through an alternative method:
javadouble[] doubleArray = {1.1, 2.2, 3.3}; String wrongStr = String.valueOf(doubleArray); // Not desirable System.out.println(wrongStr); // Outputs something like "[D@15db9742" // Correct approach using Arrays.toString() String correctStr = Arrays.toString(doubleArray); System.out.println(correctStr); // Outputs "[1.1, 2.2, 3.3]"
The example highlights that
String.valueOf()
doesn't work intuitively with non-character arrays, but usingArrays.toString()
from the Java util package does.
Conclusion
Mastering the String.valueOf()
method in Java significantly aids in converting different data types into strings, a common requirement in many programming tasks. Whether dealing with primitives, objects, or arrays, String.valueOf()
provides a smooth, exception-free way to handle conversions, even with null objects. Implement the examples shared to bolster your data handling capabilities in Java, creating more robust and error-resistant applications.
No comments yet.