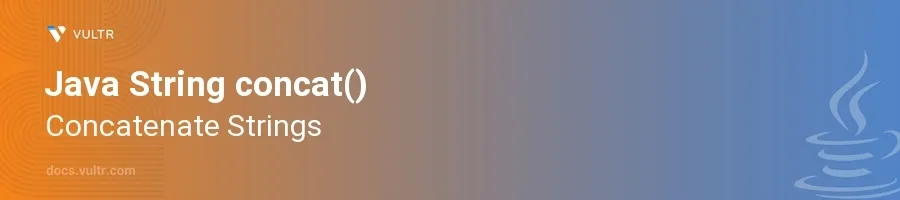
Introduction
The concat()
method in Java is designed for concatenating one string to the end of another, making it a fundamental tool for string manipulation in Java programming. This method is especially useful when you need to merge two strings to form a new string as a result.
In this article, you will learn how to efficiently utilize the concat()
method in various scenarios. Explore how this method works, understand its benefits, and see practical examples of string concatenation using concat()
.
Understanding the concat() Method
Basic Usage of concat()
Start by declaring two strings that you wish to concatenate.
Use the
concat()
method by calling it on the first string and passing the second string as an argument.javaString firstString = "Hello"; String secondString = " World!"; String result = firstString.concat(secondString); System.out.println(result);
This code snippet will output "Hello World!". The
concat()
method effectively appendssecondString
tofirstString
.
Concatenating Multiple Strings
Chain the
concat()
method to concatenate more than two strings.javaString string1 = "Java"; String string2 = " is"; String string3 = " awesome!"; String result = string1.concat(string2).concat(string3); System.out.println(result);
Here,
string1
concatenates withstring2
, and the result is further concatenated withstring3
, yielding "Java is awesome!".
Use Cases and Patterns
Concatenating Constants and Variables
Mix strings constants and variables to dynamically build messages.
javaString user = "Alice"; String greeting = "Hello, ".concat(user).concat("!"); System.out.println(greeting);
This pattern is useful for personalized user messages, combining fixed text with variable data.
Avoiding Pitfalls with Null
Be cautious of
null
values which can causeNullPointerException
.javaString nullString = null; String regularString = "Just a string"; try { String result = regularString.concat(nullString); System.out.println(result); } catch (NullPointerException e) { System.out.println("Cannot concatenate with null!"); }
This try-catch block handles potential exceptions by catching
NullPointerException
and printing a warning message.
Conclusion
The concat()
method in Java is a powerful tool for string manipulation, crucial for creating full, dynamic sentences or messages from smaller string parts. Its straightforward functionality allows for clear intentions in code, leading to better readability and maintainability. By mastering the usage of concat()
and being aware of potential pitfalls like null values, enhance your Java programs with efficient string concatenation capabilities.
No comments yet.