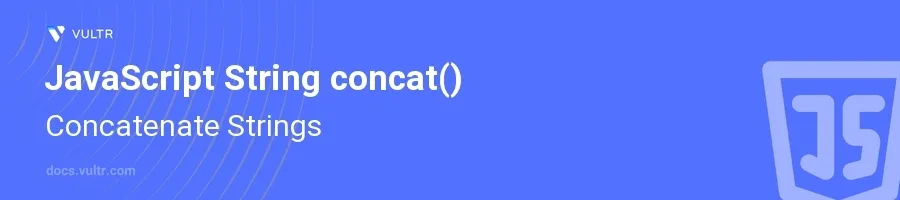
Introduction
The concat()
method in JavaScript is a straightforward approach to joining two or more strings into a single string. Valued for its simplicity and readability, concat()
simplifies string operations by eliminating the need for more complex string handling techniques such as looping or manual concatenation.
In this article, you will learn how to effectively utilize the concat()
method in various scenarios when programming in JavaScript. Discover how to leverage this method to handle strings efficiently, from appending single characters to combining multiple strings and tabs of different data types.
Basic Concatenation of Strings
Append a Single String to Another
Start with a base string.
Use the
concat()
method to append another string to it.javascriptlet baseString = "Hello"; let appendString = " World"; let combinedString = baseString.concat(appendString); console.log(combinedString);
This code appends
" World"
to"Hello"
, resulting in the output"Hello World"
.
Combine Multiple Strings
Prepare several strings to concatenate.
Apply
concat()
multiple times or in a single call to combine the strings.javascriptlet greet = "Hello"; let target = "World"; let punctuation = "!"; let fullGreeting = greet.concat(" ", target, punctuation); console.log(fullGreeting);
The
concat()
method efficiently combines "Hello", " ", "World", and "!", resulting in"Hello World!"
.
Advanced String Handling
Concatenating with Different Data Types
Recognize that
concat()
automatically converts numeric and other non-string values to strings.Combine strings with numbers and other types.
javascriptlet base = "The total is "; let number = 150; let message = base.concat(number); console.log(message);
This example concatenates a string with a number, producing
"The total is 150"
.
Handling undefined or null Values
Know that if
undefined
ornull
is concatenated, they are converted to their string representations.Concatenate strings with
undefined
andnull
safely.javascriptlet str = "Result: "; let result = undefined; let finalString = str.concat(result); console.log(finalString);
In this case, concatenating
"Result: "
withundefined
results in"Result: undefined"
.
Conclusion
The concat()
function in JavaScript offers a seamless and effective way to join strings, whether you're dealing with text, numbers, or other data types. It simplifies tasks that involve building or generating dynamic strings, thus promoting code clarity and maintainability. Apply concat()
in various contexts to enhance your string manipulation practices, ensuring your JavaScript code is clean and efficient. By mastering the techniques discussed, you'll appreciate how concat()
saves time and effort in numerous programming scenarios.
No comments yet.