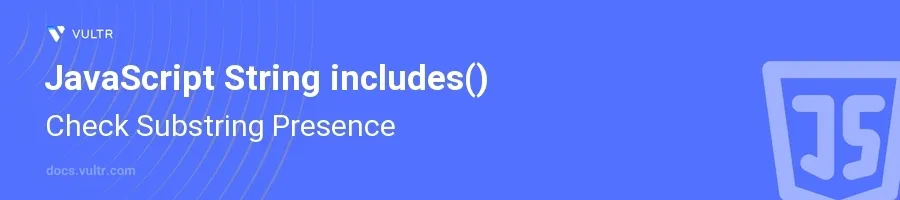
Introduction
The includes()
method in JavaScript provides a straightforward approach to verify if a string contains a specific substring. This method is essential for searching text and validating the presence of parts of strings within larger strings, enhancing string handling in various applications.
In this article, you will learn how to effectively utilize the includes()
method in different scenarios. Understand how to deploy this function to check for the presence of substrings within strings, exploring its behavior with various test cases and how it performs in case-sensitive searches.
Understanding the includes() Method
Basic Usage of includes()
Define a string variable to search within.
Use the
includes()
method to check for a particular substring.javascriptvar mainString = "Hello, world!"; var subString = "world"; var result = mainString.includes(subString); console.log(result);
This code searches for the substring
"world"
in the string stored inmainString
. Theincludes()
method returnstrue
because"world"
exists within"Hello, world!"
.
Case Sensitivity in Searches
Recognize that the
includes()
method is case-sensitive.Test the
includes()
method with different cases to see its behavior.javascriptvar text = "JavaScript is fun"; var search = "javascript"; var isPresent = text.includes(search); console.log(isPresent); // Outputs: false
In this example, the search for
"javascript"
returnsfalse
becauseincludes()
is case-sensitive and the case does not match the one intext
.
Using includes() with Position
Know that you can specify a starting position in the
includes()
method.Pass a second argument to define where to start searching in the string.
javascriptvar sentence = "Learning JavaScript is fun"; var word = "JavaScript"; var position = 9; var found = sentence.includes(word, position); console.log(found); // Outputs: true
Here, the search begins at position
9
. Since"JavaScript"
starts at that position, the method returnstrue
.
Advanced Techniques with includes()
Combining includes() with Conditional Logic
Apply the
includes()
method within a conditional statement to perform different actions based on substring presence.Use this for validations or to filter text.
javascriptvar greeting = "Hello, how are you?"; if (greeting.includes("Hello")) { console.log("The greeting is polite."); } else { console.log("The greeting is not polite."); }
This snippet checks if the greeting starts with
"Hello"
. Based on that, it logs a message about the politeness of the greeting.
Handling Multiple Conditions
Check for multiple substrings within a string using multiple
includes()
calls.Combine these checks with logical operators for complex conditions.
javascriptvar message = "Welcome to the programming world!"; var check1 = "Welcome"; var check2 = "world"; var isValid = message.includes(check1) && message.includes(check2); console.log(isValid); // Outputs: true
The code above verifies if both
"Welcome"
and"world"
are present in the message, effectively handling more than one condition.
Conclusion
The includes()
method in JavaScript is an invaluable tool for verifying the presence of substrings within strings efficiently. It helps in various programming tasks including validations, searches, and conditional functionalities. By mastering the includes()
method detailed in this discussion, your handling of strings in JavaScript will be more precise and suited to the needs of diverse applications. Explore the capabilities of this method to enhance your programming projects significantly.
No comments yet.