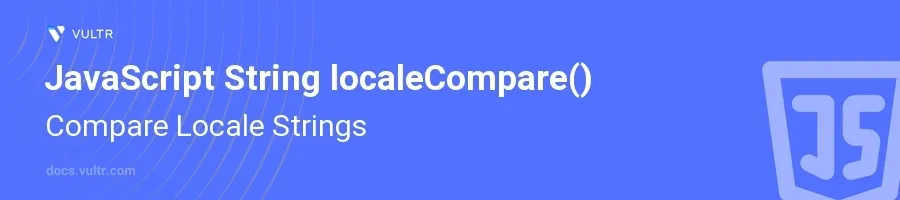
Introduction
The localeCompare()
method in JavaScript offers a powerful solution for comparing two strings in the current or specified locale—an essential tool in internationalization. By directly considering locale-specific rules, such as alphabet order or accents, this functionality enhances the software's ability to operate across different languages and regional data formats.
In this article, you will learn how to effectively utilize the localeCompare()
method for various scenarios where locale-sensitive string comparison is necessary. Understand how to deploy this method to sort an array of strings, compare specific linguistic rules, and even customize comparisons using locales and options.
Using localeCompare() for Basic Comparisons
Compare Two Strings in the Current Locale
Start by defining two strings in any language.
Use the
localeCompare()
method to compare these strings and obtain a result that signifies their relative order.javascriptlet str1 = "apple"; let str2 = "banana"; let result = str1.localeCompare(str2); console.log(result);
This code compares
str1
withstr2
using the current locale settings. The method returns-1
,0
, or1
depending on whetherstr1
comes before, is the same as, or comes afterstr2
lexicographically.
Sort an Array of Strings
Define an array of strings.
Use the
sort()
method incorporatinglocaleCompare()
to accurately sort the array based on local conventions.javascriptlet fruits = ["cherry", "banana", "apple"]; fruits.sort((a, b) => a.localeCompare(b)); console.log(fruits);
This snippet sorts an array of English words in alphabetical order according to the rules of the locale. Note how
localeCompare()
is used as a comparison function withinsort()
.
Advanced Usage of localeCompare()
Customizing with Locales and Options
Recognize that
localeCompare()
can take additional parameters to specify locales and sorting options.Specify a locale and options such as
sensitivity
to achieve different comparison behaviors.javascriptlet str1 = "Straße"; let str2 = "Strasse"; let locale = "de-DE"; // German locale let options = { sensitivity: 'base' }; let result = str1.localeCompare(str2, locale, options); console.log(result);
Here, strings are compared based on the German locale. The
sensitivity: 'base'
option means that differences in case and accents are ignored, which influences the comparison outcome.
Handling Multiple Locale Scenarios
Consider scenarios where users might have different regional settings.
Use a mix of locales and compare how
localeCompare()
behaves differently with each setting.javascriptlet str1 = "resume"; let str2 = "résumé"; let results = []; results.push(str1.localeCompare(str2, 'en-US')); results.push(str1.localeCompare(str2, 'fr-FR')); console.log(results);
This example assesses the comparison results using both American English and French rules. Depending on the locale, 'resume' might or might not be considered equal to 'résumé', reflecting locale-specific behavior.
Conclusion
The localeCompare()
method in JavaScript bridges the critical gap in performing locale-sensitive string comparisons, vital for applications serving a global audience. Mastering this function allows for precise control over how textual data is compared and sorted, considering the unique linguistic and cultural norms of different regions. Implement these techniques to ensure your applications respect locale nuances, leading to more intuitive and effective international functionality.
No comments yet.