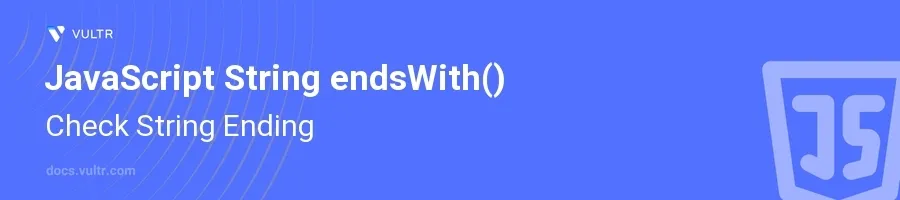
Introduction
The endsWith()
method in JavaScript is a straightforward and efficient way to determine whether a string ends with specific characters or a substring. This method is especially useful in scenarios where you need to validate or assess string data based on its ending pattern, such as file extensions, certain protocols, suffixes, and more.
In this article, you will learn how to use the endsWith()
method in various contexts. Explore different examples to understand how this method operates with direct strings, variables, and its utilization with conditional statements for effective string analysis and validation.
Using endsWith() to Check String Endings
Basic Usage of endsWith()
Start with a basic string.
Use the
endsWith()
method to check if the string ends with a specified substring.javascriptlet message = "Hello, world!"; let checkEnding = message.endsWith("world!"); console.log(checkEnding);
In this code,
checkEnding
will betrue
because the string inmessage
ends with"world!"
.
Case Sensitivity
Remember that
endsWith()
is case-sensitive.Try checking the ending with a different case to illustrate sensitivity.
javascriptlet greeting = "Hello, World!"; let result = greeting.endsWith("world!"); console.log(result);
This code outputs
false
because"World!"
is not the same as"world!"
due to different cases in the substring.
Using Variables for Substring
Use a variable to specify the substring for flexibility and dynamic checks.
Implement
endsWith()
with a variable holding the substring.javascriptlet fileName = "example.pdf"; let extension = "pdf"; let isValidExtension = fileName.endsWith(extension); console.log(isValidExtension);
Here,
isValidExtension
will returntrue
, demonstrating how variables can be used to check the end of strings dynamically.
Combining with Conditional Logic
Incorporate
endsWith()
within if-else structures for enhanced decision-making.Set up conditions based on the ending of a string.
javascriptlet url = "https://example.com/data.json"; if (url.endsWith(".json")) { console.log("The URL points to a JSON file."); } else { console.log("The URL does not point to a JSON file."); }
This control flow checks if
url
ends with".json"
; if true, it confirms that the URL points to a JSON file.
Conclusion
The endsWith()
method in JavaScript is essential for validating the ending segment of strings in various applications. Whether checking file extensions, managing URL formats, or ensuring consistency in user inputs, endsWith()
enhances the robustness and reliability of string handling in JavaScript applications. Equip your toolkit with this method to streamline tasks involving string analysis and ensure data integrity through simple yet effective validation checks.
No comments yet.