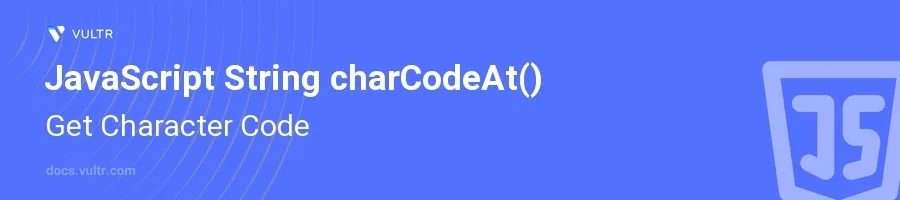
Introduction
The charCodeAt()
method in JavaScript is a powerful string function used to retrieve the Unicode value of a character at a specified position in a string. This method serves as an essential tool for those involved in tasks like encoding, decoding, or even validation of data where character encoding plays a crucial role.
In this article, you will learn how to effectively utilize the charCodeAt()
method in various situations. Discover how to access character codes in strings, handle various positions within strings, and see practical examples that demonstrate the method's utility in day-to-day coding.
Understanding charCodeAt()
Retrieve Unicode Value of a Character
Access a single character's Unicode value by specifying its index.
Use the
charCodeAt()
method on a string.javascriptconst message = "Hello, World!"; const index = 7; const charCode = message.charCodeAt(index); console.log(charCode);
In this example, the
charCodeAt()
method retrieves the Unicode value for the character at index7
, which isW
. The output will be87
, the Unicode value forW
.
Handling Characters at Different Positions
Iterate over a string to get the Unicode values of all characters.
Apply
charCodeAt()
in a loop to access each character's code.javascriptconst phrase = "Hello!"; for(let i = 0; i < phrase.length; i++) { console.log(`Character: ${phrase[i]}, Code: ${phrase.charCodeAt(i)}`); }
This script prints the Unicode value for each character in the string
"Hello!"
. It iterates through each position, usingcharCodeAt()
to obtain each character's code.
Edge Case: No Index Provided
Understand that
charCodeAt()
defaults to the first character if no index is provided.Observe how the method behaves when called without arguments.
javascriptconst greeting = "Hello"; const defaultCharCode = greeting.charCodeAt(); console.log(defaultCharCode);
If no index is provided,
charCodeAt()
assumes the index to be0
. For the string"Hello"
, it returns72
, which is the Unicode value ofH
.
Practical Uses of charCodeAt()
Validating Input
Use
charCodeAt()
to ensure that a string contains characters from a specific range of Unicode values.Apply it in form validation where character restrictions are essential.
javascriptfunction isAlphaNumeric(input) { for (let i = 0; i < input.length; i++) { const code = input.charCodeAt(i); if (!(code > 47 && code < 58) && // numeric (0-9) !(code > 64 && code < 91) && // upper alpha (A-Z) !(code > 96 && code < 123)) { // lower alpha (a-z) return false; } } return true; } const userInput = "Hello123"; console.log(isAlphaNumeric(userInput)); // Output: true
This function checks whether the input string consists only of alphanumeric characters by comparing character codes against known ranges for numeric and alphabetic characters.
Conclusion
The charCodeAt()
function in JavaScript is invaluable for handling operations involving character encoding. It offers a straightforward way to retrieve Unicode character codes, thus facilitating many tasks from data validation to intricate text processing. By mastering charCodeAt()
, you enhance the capability to manage string manipulations involving character codes proficiently. Whether validating input or encoding data, this tool helps maintain robust and effective JavaScript applications.
No comments yet.