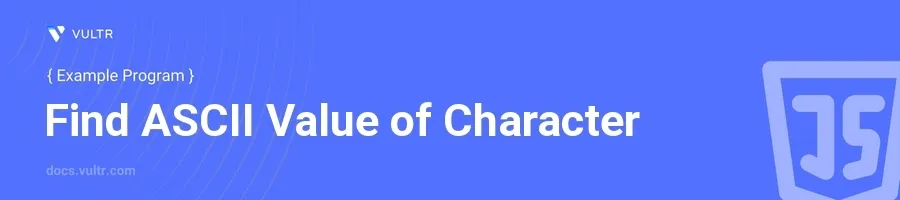
Introduction
Finding the ASCII value of a character is a common task in programming that can be useful for various operations such as sorting, data manipulation, and encryption. ASCII (American Standard Code for Information Interchange) assigns a unique decimal number to different characters and symbols, which computers use to represent text.
In this article, you will learn how to find the ASCII value of a character in JavaScript. These examples will guide you through different scenarios to demonstrate the versatility and functionality of handling ASCII values in your scripts.
Finding ASCII Value of a Single Character
Using the charCodeAt()
Method
Identify the character you wish to find the ASCII value for.
Use the JavaScript string method
charCodeAt()
to find the ASCII value.javascriptlet character = 'A'; let asciiValue = character.charCodeAt(0); console.log(asciiValue);
In this example,
charCodeAt(0)
is used to find the ASCII value of the first (and only) character in the stringcharacter
. It prints65
, which is the ASCII value for 'A'.
Handling Non-Standard Characters
Choose a non-standard or extended character such as 'ñ' or '€'.
Apply the
charCodeAt()
method as well.javascriptlet character = 'ñ'; let asciiValue = character.charCodeAt(0); console.log(asciiValue);
Here, the ASCII value for 'ñ' is
241
. Note that extended ASCII values like this may vary depending on the character encoding.
Generalizing to Multiple Characters
Iterating Over a String
Create a string containing multiple characters.
Loop through each character in the string to print their ASCII values.
javascriptlet text = "Hello"; for (let i = 0; i < text.length; i++) { let asciiValue = text.charCodeAt(i); console.log(`ASCII of ${text[i]}: ${asciiValue}`); }
This code snippet loops through the string
text
and prints out the ASCII value for each character. It helps in visually identifying the values for comparative or analytical purposes.
Conclusion
Extracting ASCII values in JavaScript is simplified with the use of the charCodeAt()
method. Knowing how to retrieve these values is essential for tasks that require precise character handling such as creating hash keys, encoding, and many other programming tasks where character data are crucial. With the knowledge gained, you'll be able to manipulate and utilize character data more effectively in your JavaScript projects.
No comments yet.