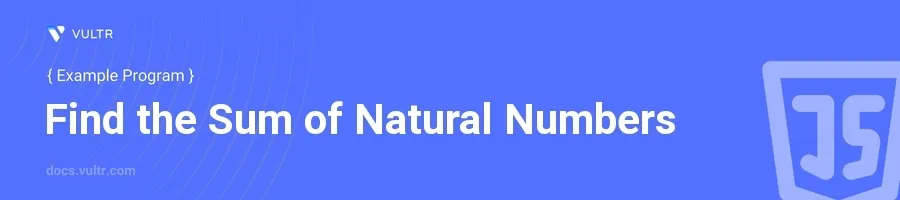
Introduction
Working with natural numbers is a fundamental aspect of programming, often required in various computational problems and algorithms. In JavaScript, you can implement simple yet efficient logic to compute the sum of natural numbers using different methods. This computation might seem straightforward but serves as a good practice for understanding loops and arithmetic operations in JavaScript.
In this article, you will learn how to find the sum of natural numbers using JavaScript. Discover different methods to accomplish this task, including using a for loop and the mathematical formula for the sum of an arithmetic series. Each method will be highlighted with code examples to help you understand and implement the solution effectively.
Using a For Loop
Calculating Sum with Iteration
Initialize a variable to store the sum.
Use a for loop to iterate through each natural number up to the desired number.
Add each number to the sum during each iteration.
javascriptfunction sumNaturalNumbers(n) { let sum = 0; for (let i = 1; i <= n; i++) { sum += i; } return sum; } console.log(sumNaturalNumbers(100)); // 5050
In this code,
sumNaturalNumbers
is a function that computes the sum of all natural numbers up ton
. The loop iteratively adds each number from 1 ton
tosum
, accumulating the total sum by the end of the loop. This example calculates the sum of the first 100 natural numbers.
Utilizing Mathematical Formula
Direct Computation Using Formula
Use the formula ( \text{Sum} = \frac{n \times (n + 1)}{2} ) to calculate the sum without a loop.
Implement this formula within a function to compute the sum instantly.
javascriptfunction sumNaturalNumbersFormula(n) { return (n * (n + 1)) / 2; } console.log(sumNaturalNumbersFormula(100)); // 5050
The formula ( n \times (n + 1) / 2 ) directly computes the sum of the first
n
natural numbers. This method is much more efficient than using a loop, especially for a large numbern
.
Conclusion
The sum of natural numbers can be calculated in JavaScript using either an iterative approach with a for loop or a direct mathematical formula. The iterative method gives you a deeper understanding of loops and incremental summing, whereas the formula method provides a quick and efficient way to compute the sum for large values. Depending on the situation and the scale of the numbers involved, choose the approach that best suits your needs. Integrate these methods into larger programs where summing natural numbers is required, ensuring your solutions are both effective and optimal.
No comments yet.