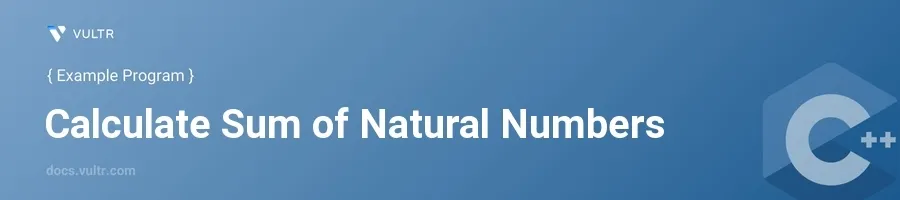
Introduction
Calculating the sum of natural numbers is a classic exercise in programming, particularly for those who are new to the language or to programming in general. This concept often serves as a bridge to understanding loops and conditional statements in C++, which are foundational concepts for any programmer.
In this article, you will learn how to efficiently calculate the sum of natural numbers using C++. We will explore different methods, including using a for-loop, the formula for the sum of the first 'n' natural numbers, and using recursion. Each approach serves different needs and illustrates key aspects of programming in C++.
Using a For Loop
Sum Calculation with Incrementing Values
Start by declaring and initializing variables. A variable for storing the sum (
sum
) is initialized to 0, and another variable (n
) is used to store the upper limit input by the user.Implement a for-loop that iterates from 1 to
n
, adding the loop variable to the sum during each iteration.cpp#include <iostream> using namespace std; int main() { int n, sum = 0; cout << "Enter the number of natural numbers: "; cin >> n; for (int i = 1; i <= n; i++) { sum += i; } cout << "Sum of the first " << n << " natural numbers is: " << sum << endl; return 0; }
This code snippet initializes
sum
to 0 and iterates from 1 ton
, cumulatively addingi
tosum
. When the loop completes, the sum of the firstn
natural numbers is printed.
Using the Formula Method
Direct Calculation Using a Mathematical Approach
Use the mathematic formula for the sum of the first
n
natural numbers: ( \frac{n(n + 1)}{2} ).Implement this formula in a function to make it reusable.
cpp#include <iostream> using namespace std; int sumOfNaturalNumbers(int n) { return n * (n + 1) / 2; } int main() { int n; cout << "Enter the number of natural numbers: "; cin >> n; cout << "Sum of the first " << n << " natural numbers is: " << sumOfNaturalNumbers(n) << endl; return 0; }
This program defines the function
sumOfNaturalNumbers(int n)
, which calculates and returns the sum of the firstn
natural numbers using the formula ( \frac{n(n + 1)}{2} ). The function is called after the user inputs the value ofn
.
Using Recursion
Recursive Function for Sum Calculation
Define a recursive function that calls itself until it reaches the base case, where the number decreases to zero.
In each recursive call, add the current number to the result of the recursive call using the number decremented by one.
cpp#include <iostream> using namespace std; int recursiveSum(int n) { if (n == 0) return 0; return n + recursiveSum(n - 1); } int main() { int n; cout << "Enter the number of natural numbers: "; cin >> n; cout << "Sum of the first " << n << " natural numbers is: " << recursiveSum(n) << endl; return 0; }
In this snippet, the function
recursiveSum(int n)
calculates the sum of the firstn
natural numbers by recursively calling itself with the decremented value ofn
. The recursion stops whenn
is 0, at which point it starts unwinding and cumulative adding the values ofn
.
Conclusion
Calculating the sum of natural numbers is a versatile task that can be approached in multiple ways in C++. Whether you prefer the iterative strength of a for-loop, the mathematical shortcut using a formula, or the elegance of a recursive function, each method has its own merits. Mastering these techniques not only helps in solving this specific problem but also enhances understanding of key programming concepts in C++. Use these approaches to further refine your problem-solving skills and apply them in various computing scenarios.
No comments yet.