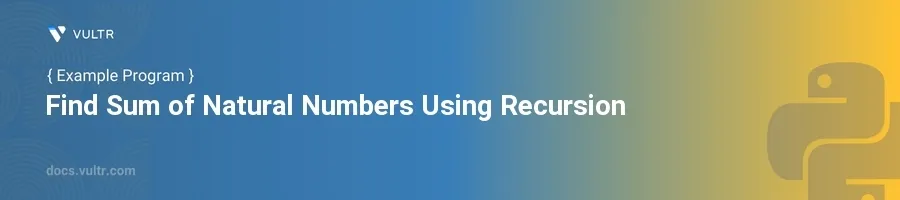
Introduction
Recursion in programming refers to a function calling itself in its definition. This elegant coding technique is not just theoretical but immensely useful, especially when solving problems that can be broken down into similar subproblems. One classic example is calculating the sum of natural numbers, where the sum of first n
natural numbers is the sum of n
plus the sum of numbers before n
.
In this article, you will learn how to harness recursion to compute the sum of natural numbers in Python. Explore practical, clear examples that enhance your understanding of recursive functions and demonstrate how they provide solutions to typical mathematical problems. By the end, master creating a recursive function that calculates the sum of first n
natural numbers efficiently.
Understanding Recursion
Recursion involves a function calling itself with modified parameters until it reaches a stopping condition, known as the base case. In the context of summing natural numbers, the general idea is to have the function call itself with the next smaller number until it reaches 1. Here's a step-by-step guide to thinking recursively for summing natural numbers:
Determine the Basic Case
- Identify the simplest possible input for which the answer is known without any further recursion. For the sum of natural numbers, if
n
is 1, then the sum is obviously 1.
Define the Reduction Step
- Decide how to reduce the problem size with each function call. For summing natural numbers, this involves calling the same function with
n - 1
untiln
reaches 1.
Implement Recursion
- Combine these ideas into a function that calls itself, reducing the problem size, and accumulates the result.
Recursive Function to Calculate Sum
Let's implement the recursive approach to calculate the sum of natural numbers.
Code Implementation
- First, define the function
sum_of_natural_numbers
that accepts a single parameter,n
, representing the highest number in the range of natural numbers to sum. - Next, set up the base case to stop the recursion when
n
equals 1. - For the reduction step, if
n
is greater than 1, call the function itself withn - 1
and addn
to the result of this function call.
def sum_of_natural_numbers(n):
if n == 1:
return 1
else:
return n + sum_of_natural_numbers(n-1)
Code Explanation
- When
n == 1
, the function returns 1, as the sum of the first natural number is trivially 1. This acts as the base case preventing further recursion. - If
n
is greater than 1, the function continues to addn
to the result ofsum_of_natural_numbers(n-1)
, reducingn
each time it is called recursively, eventually reaching the base case.
Demonstration with Examples
- Calculate the sum of the first 5 natural numbers.
- Calculate the sum of the first 10 natural numbers.
Run the above code, and view the outputs:
print(sum_of_natural_numbers(5)) # Output should be 15
print(sum_of_natural_numbers(10)) # Output should be 55
Conclusion
Utilizing recursion in Python to solve the problem of summing natural numbers is an excellent way to practice thinking in terms of self-referential functions. This technique not only simplifies problems that can be divided into similar smaller chunks but also promotes a more profound understanding of function operations and stack usage in programming. Through the explanations and examples presented, you now possess the ability to deploy recursion for both simple arithmetic tasks and potentially more complex algorithmic challenges. Explore further how recursion can be utilized in various other programming scenarios to enhance your coding efficiency and problem-solving skills.
No comments yet.