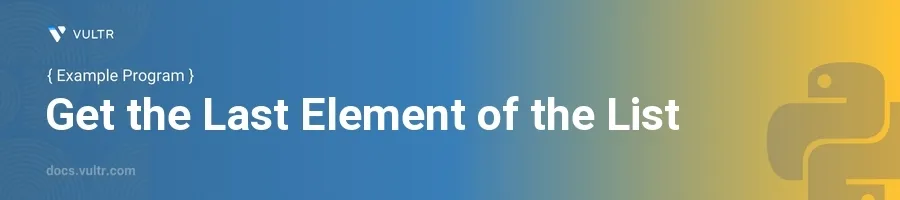
Introduction
Reaching the terminal point of a list signals the conclusion of a collection but often, the last element holds specific significance whether in computations, validation processes, or just retrieving the last logged item. Handling lists efficiently in Python forms a cornerstone for data manipulation tasks across various applications.
In this article, you will learn how to effectively extract the last element from a list in Python. Explore different methods, each tailored to accommodate various programming scenarios such as handling standard lists, dealing with empty lists, and optimizing code performance for larger datasets.
Extracting the Last Element Directly
Utilizing Negative Indexing
Recognize that Python lists support negative indexing, where -1 refers to the last element.
Retrieve the last element of a non-empty list using negative indexing.
pythonitems = [2, 5, 7, 9] last_item = items[-1] print(last_item)
The output will display
9
. This method is the most straightforward when you know the list contains at least one element.
Validating List Content
Check if the list is non-empty before accessing its content.
Use an if statement to prevent errors such as an
IndexError
.pythonitems = [] if items: print(items[-1]) else: print("The list is empty.")
This approach ensures error-free execution by handling empty lists effectively.
Leveraging Python Built-in Functions
Using the list.pop() Method
Understand that
list.pop()
not only retrieves but also removes the last element from the list.This function is useful when you need to retrieve the last element and no longer need it in the list.
pythonitems = [3, 8, 12] last_item = items.pop() print(last_item)
This code will output
12
, and the listitems
will be updated to[3, 8]
.
Applying list slicing
Recognize the utility of list slicing for accessing elements.
Employ slicing to obtain the last element without modifying the original list.
pythonitems = [1, 4, 6, 10] last_item = items[-1:] print(last_item[0])
Although this code segment uses slicing to obtain the last element as a list containing only that element, it is less direct than negative indexing.
Handling Larger Lists
Employing itertools for Efficiency
Admit the importance of efficient data handling with large datasets.
Use
itertools.islice
to efficiently retrieve the last element when working with extremely large lists.pythonimport itertools items = range(1000000) last_item = next(itertools.islice(items, 999999, None)) print(last_item)
This example displays
999999
, demonstrating an efficient approach for large data sequences by avoiding loading the entire list into the memory.
Conclusion
The capability to fetch the last item from a list in Python enhances flexibility in data manipulation and is essential for various practical applications ranging from data completion to state checks in looped processes. Utilizing the techniques discussed, such as negative indexing, built-in functions like pop()
, or even itertools
for efficiency, you can adapt your approach based on the context and requirements of the task at hand, ensuring your code remains efficient and easy to manage. Whether handling small or large data sets, these methods provide robust solutions for dealing with end elements of lists effectively.
No comments yet.