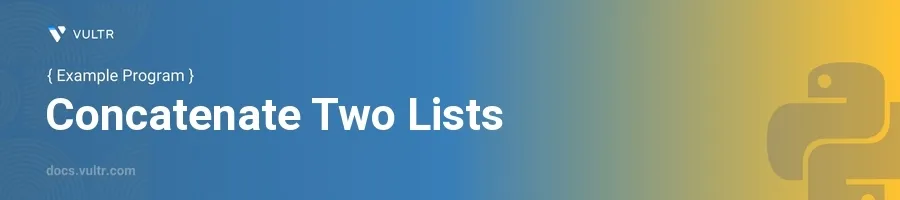
Introduction
Concatenating lists in Python is a fundamental and frequently used operation. It refers to the process of combining two or more lists into a single list. This is widely applied in data manipulation, sequence processing, and in any scenario where you need to merge data from different sources into one unified structure.
In this article, you'll learn how to concatenate two lists in Python using various techniques. Whether you're looking to combine two lists in Python, merge two lists, or append two lists, these methods cover everything from simple operations to more advanced approaches using built-in libraries.
Basic List Concatenation in Python
Using the +
Operator
Define two lists that you want to concatenate.
Use the
+
operator to combine them into a single list.pythonlist1 = [1, 2, 3] list2 = [4, 5, 6] concatenated_list = list1 + list2 print(concatenated_list)
This method adds two lists in Python and creates a new one with the elements of both lists. In our code, it is adding
list2
to the end oflist1
, resulting in a new list[1, 2, 3, 4, 5, 6]
.
Using the extend()
Method
Use an existing list.
Apply the
extend()
method with another list as the argument to append its elements.pythonlist1 = [1, 2, 3] list2 = [4, 5, 6] list1.extend(list2) print(list1)
The
extend()
method modifieslist1
in-place by appending elements fromlist2
, resulting inlist1
being[1, 2, 3, 4, 5, 6]
. This is useful when you want to directly concatenate a Python list without creating a new one.
Advanced Concatenation Techniques in Python
Using List Comprehension
Create a new list by iterating over elements of both lists sequentially.
This method is ideal for concatenating a list of lists in Python that may involve complex conditions or transformations.
pythonlist1 = [1, 2, 3] list2 = [4, 5, 6] concatenated_list = [item for sublist in [list1, list2] for item in sublist] print(concatenated_list)
This list comprehension flattens the structure and joins both lists in Python while also supporting customization if needed.
Using itertools.chain()
Import the
itertools
module.Use the
chain()
function to concatenate the lists in Python.pythonfrom itertools import chain list1 = [1, 2, 3] list2 = [4, 5, 6] concatenated_list = list(chain(list1, list2)) print(concatenated_list)
The
chain()
function from theitertools
module concatenates multiple lists, which is especially useful when working with more than two lists or larger datasets.
Conclusion
Understanding how to concatenate lists in Python is essential for effective data manipulation. Python provides multiple ways to merge two lists or append one list to another using methods like the +
operator, extend()
, list comprehension, and itertools.chain()
.
By mastering these approaches, you’ll know how to combine two lists in Python, whether you’re working with flat lists or nested structures. This flexibility makes Python powerful for handling list operations in diverse programming scenarios.
No comments yet.